Rendering Active¶
In this guide you will learn how to use switch rendering
of the interactive MapView
on and off; when rendering is off,
the map is not updated and the view appears frozen regardless of
user input.
Freeze rendering and toggle fps stats¶
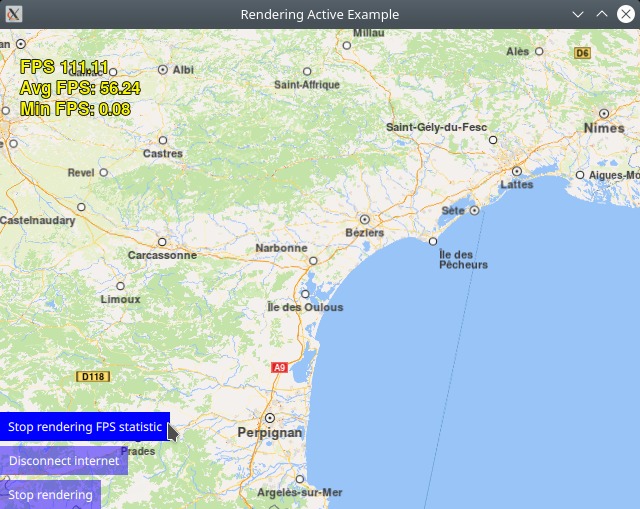
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
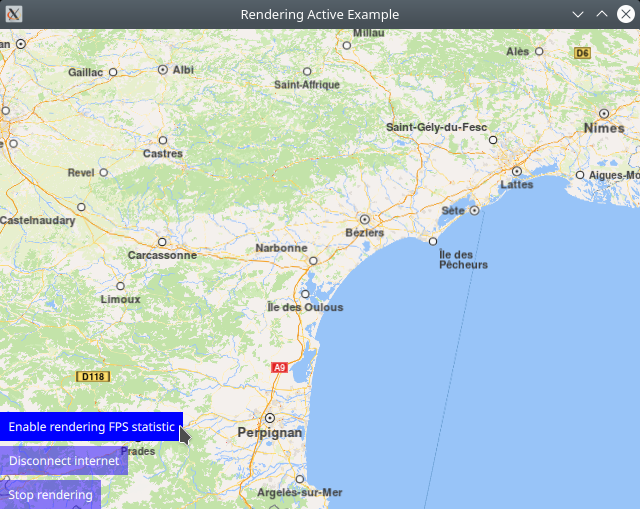
RenderingActive
demonstrates how easy it is to use MapView
to render an interactive map, and using the rendering
property to
switch rendering on and off.
Drawing fps (frames per second) and other camera statistics on-screen,
as well as switching the internet connection on and off,
are also demonstrated.
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the RenderingActive example folder and open RenderingActive.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
In main.qml, we import the GeneralMagic QML plugin. Next, we set
ServicesManager.settings.allowInternetConnection = true
,
which means the network connection is active, and thus
maps, voices and map styles can be downloaded.
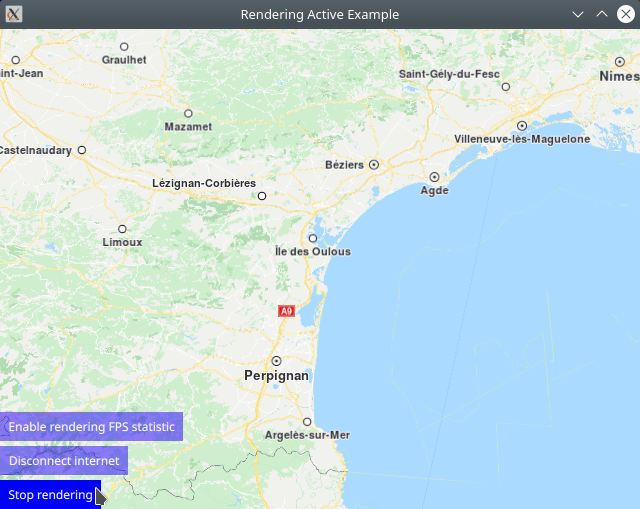
1import QtQuick 2.12
2import QtQuick.Controls 2.12
3import QtQuick.Layouts 1.12
4//! [Rendering wiring]
5import QtQuick.Window 2.12
6import GeneralMagic 2.0
7
8Window {
9 visible: true
10 width: 640
11 height: 480
12 title: qsTr("Rendering Active Example")
13
14 property var updater: ServicesManager.contentUpdater(ContentItem.Type.RoadMap)
15 Component.onCompleted: {
16 //! [Set token safely]
17 ServicesManager.settings.token = __my_secret_token;
18 //! [Set token safely]
19 ServicesManager.settings.allowInternetConnection = true; // enable connection to online services
20
21 mapView.onDrawFPSChanged();
22 mapView.update();
23
24 updater.autoApplyWhenReady = true;
25 updater.update();
26 }
27//! [Rendering wiring]
Toggle map rendering¶
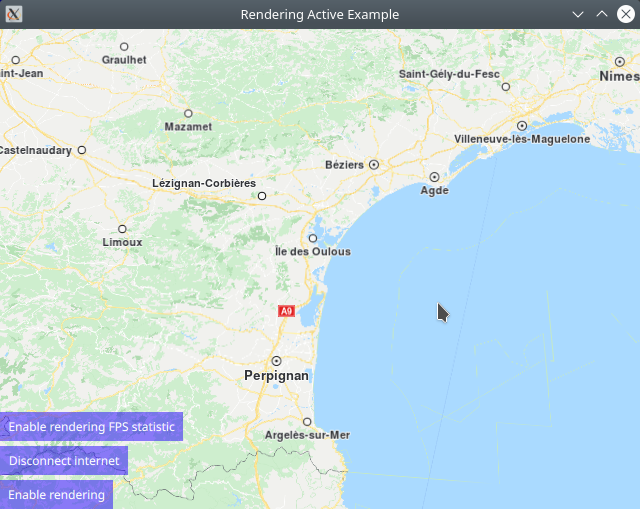
An interactive map is displayed using MapView
and
a button to toggle on-screen fps and camera statistics on/off,
a button to toggle the internet connection on/off,
which affects whether maps, voices and map styles can be downloaded, along with
a button to toggle map rendering on/off, are in the lower left corner.
1MapView {
2 id: mapView
3 anchors.fill: parent
4 viewAngle: 25
5 cursorVisibility: false
6 drawFPS: true
7 onDrawFPSChanged: {
8 console.log("Draw FPS was toggled to " + mapView.drawFPS);
9 fpsToggle.text = mapView.drawFPS ? "Stop rendering FPS statistic" : "Enable rendering FPS statistic"
10 mapView.update();
11 }
12
13 ColumnLayout {
14 anchors.left: parent.left
15 anchors.bottom: parent.bottom
16 Button {
17 id: fpsToggle
18 text: "Enable rendering FPS statistic"
19 background: Rectangle {
20 opacity: parent.hovered ? 1 : 0.5
21 color: enabled ? parent.down ? "#aa00aa" :
22 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
23 }
24 palette { buttonText: "#ffffff"; }
25 onClicked: {
26 mapView.drawFPS = !mapView.drawFPS;
27 }
28 }
29 Button {
30 text: ServicesManager.settings.allowInternetConnection ? "Disconnect internet" : "Connect internet"
31 background: Rectangle {
32 opacity: parent.hovered ? 1 : 0.5
33 color: enabled ? parent.down ? "#aa00aa" :
34 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
35 }
36 palette { buttonText: "#ffffff"; }
37 onClicked:
38 // enable connection to online services
39 ServicesManager.settings.allowInternetConnection = !ServicesManager.settings.allowInternetConnection;
40 }
41 Button {
42 text: mapView.rendering ? "Stop rendering" : "Enable rendering"
43 background: Rectangle {
44 opacity: parent.hovered ? 1 : 0.5
45 color: enabled ? parent.down ? "#aa00aa" :
46 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
47 }
48 palette { buttonText: "#ffffff"; }
49 onClicked: mapView.rendering = !mapView.rendering;
50 }
51 }
52}