Text Search¶
In this guide you will learn how to search for anything on the map with a free-form text query.
See the Reverse Geocoding guide on how to use reverse geocoding search to find the positions of points of interest (POIs) near a programmatically or interactively/visually specified position on the map.
See the Search Example guide on how to perform an assisted address search.
Free Text Search¶
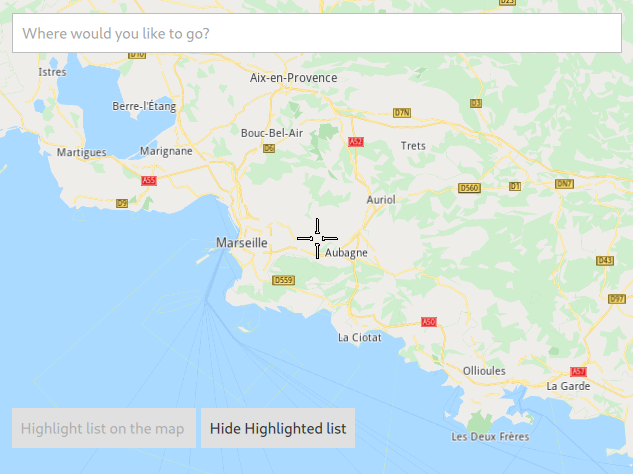
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
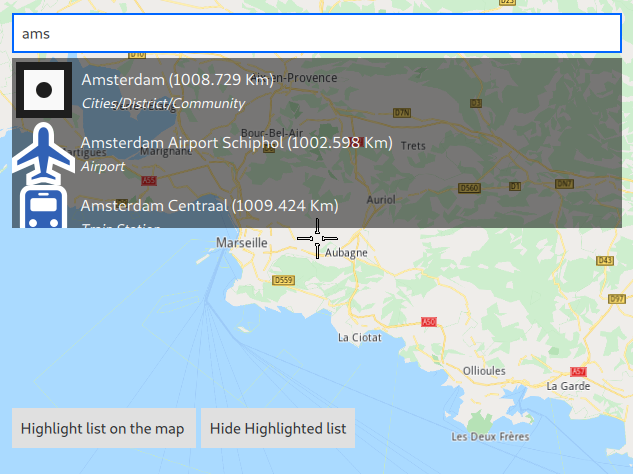
FreeTextSearch
demonstrates how to use the SearchService
to perform a
free text search. This example presents an application window displaying a map.
At the top of the window is a search box, which is used to enter a search query. To search, enter a search term into the text box. The results will be shown in the list below.
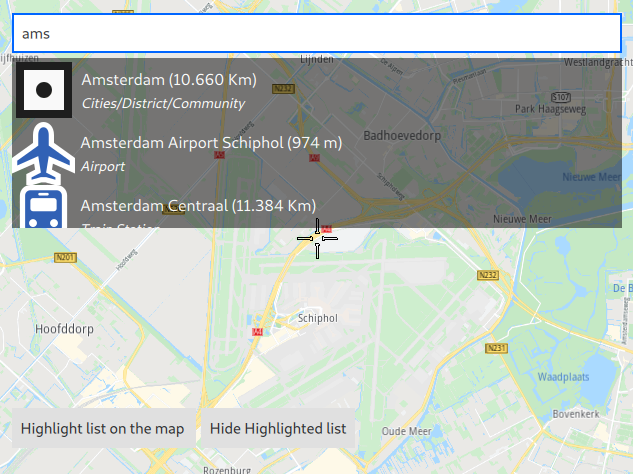
Click on any result to fly to its position.
How it works
In Qt, go to the File menu and select Open File or Project…
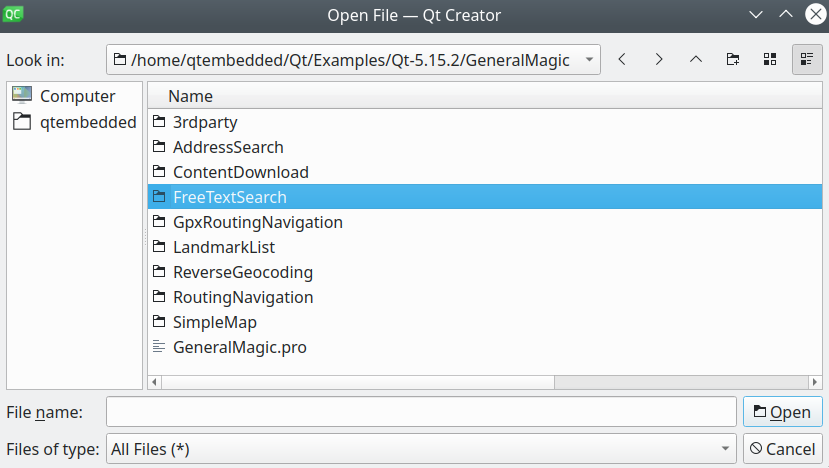
then browse to the FreeTextSearch example folder and open FreeTextSearch.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
In main.qml, we need to import the GeneralMagic QML plugin. Next, we need to make sure we allow online access, and that we are using the latest data.
1import QtQuick.Window 2.12
2import GeneralMagic 2.0
3
4Window
5{
6 visible: true
7 width: 640
8 height: 480
9 title: qsTr("Free Text Search Example")
10 Component.onCompleted:
11 {
12 ServicesManager.settings.token = __my_secret_token;
13
14 ServicesManager.settings.allowInternetConnection = true;
15 var updater = ServicesManager.contentUpdater(ContentItem.Type.RoadMap);
16 updater.autoApplyWhenReady = true;
17 updater.update();
18 }
The __my_secret_token property in the above QML code is set in C++ like this.
1// C++ code
2QQmlApplicationEngine engine;
3//...
4//! [Set API Key token safely]
5// go to https://developer.magiclane.com to get your token
6engine.rootContext()->setContextProperty("__my_secret_token", "YOUR_TOKEN");
7//! [Set API Key token safely]
8
9engine.load(url);
In this example, in main.cpp, replace YOUR_TOKEN with your actual Magic Lane Maps API Key.
We need to declare a SearchService
model to perform a search.
1SearchService
2{
3 id: searchService
4 filter: searchBar.text
5 preferences
6 {
7 searchMapPOIs: true
8 searchAddresses: true
9 limit: 10
10 }
11
12 function searchNow()
13 {
14 searchTimer.stop();
15 cancel();
16 preferences.referencePoint = mapView.cursorWgsPosition();
17 search();
18 }
19}
After that, we use an interactive text field to input the search term.
1TextField
2{
3 id: searchBar
4 Layout.fillWidth: true
5 placeholderText: qsTr("Where would you like to go?")
6 onTextChanged: searchTimer.restart()
7 onEditingFinished: searchService.searchNow()
8}
Also a timer is needed to avoid flooding the search service with search terms too often.
1Timer
2{
3 id: searchTimer
4 interval: 500
5 onTriggered:
6 {
7 searchService.searchNow();
8 }
9}
And finally we are going to put everything in a list.
1ListView
2{
3 id: searchList
4 anchors.fill: parent
5 clip: true
6 model: searchService
7 function distance(meters)
8 {
9 return meters >= 1000 ? (meters / 1000.).toFixed(3)
10 + " Km" : meters.toFixed(0) + " m";
11 }
12 delegate: Item
13 {
14 height: row.height
15 RowLayout
16 {
17 id: row
18 IconView
19 {
20 iconSource: landmark.icon
21 Layout.maximumHeight: row.height
22 Layout.maximumWidth: row.height
23 width: height
24 height: row.height
25 }
26 ColumnLayout
27 {
28 Layout.fillHeight: true
29 Layout.fillWidth: true
30 Text
31 {
32 Layout.fillWidth: true
33 text: landmark.name + " (" + searchList.distance(
34 landmark.coordinates.distance(
35 searchService.preferences.referencePoint)) + ")"
36 color: "white"
37 font.pixelSize: 16
38 wrapMode: Text.WrapAnywhere
39 }
40 Text
41 {
42 Layout.fillWidth: true
43 text: landmark.description
44 color: "white"
45 font.pixelSize: 14
46 font.italic: true
47 wrapMode: Text.WrapAnywhere
48 }
49 }
50 }
51 MouseArea
52 {
53 anchors.fill: row
54 onClicked:
55 {
56 mapView.centerOnCoordinates(
57 searchService.get(index).coordinates, -1);
58 searchBar.focus = true;
59 }
60 }
61 }
62}