Fragment Map¶
In this guide you will learn how to render
an interactive map centered on a desired location.
The map is fully 3D, supporting pan, pinch-zoom,
rotate and tilt.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the Maps & Navigation SDK for Android archive file
Download the HelloFragment project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
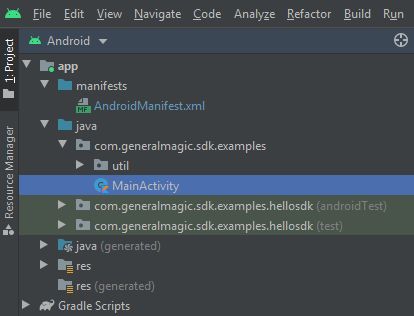
You can open the MainActivity.kt file to see how an interactive map is rendered in a fragment.
1class FirstFragment : Fragment() {
2 override fun onCreateView(
3 inflater: LayoutInflater, container: ViewGroup?,
4 savedInstanceState: Bundle?
5 ): View? {
6 return inflater.inflate(R.layout.fragment_first, container, false)
7 }
8 override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
9 super.onViewCreated(view, savedInstanceState)
10 view.findViewById<Button>(R.id.button_first).setOnClickListener {
11 findNavController().navigate(R.id.action_FirstFragment_to_SecondFragment)
12 }
13 }
14}
The first fragment.
1class SecondFragment : Fragment() {
2 override fun onCreateView(
3 inflater: LayoutInflater, container: ViewGroup?,
4 savedInstanceState: Bundle?
5 ): View? {
6 return inflater.inflate(R.layout.fragment_second, container, false)
7 }
8 override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
9 super.onViewCreated(view, savedInstanceState)
10 view.findViewById<Button>(R.id.button_second).setOnClickListener {
11 findNavController().navigate(R.id.action_SecondFragment_to_FirstFragment)
12 }
13 }
14}
The second fragment.
Each of the 2 fragments has a button to navigate to the other fragment,
see
res/navigation/nav_graph.xml
in the project.The
SecondFragment
also renders a map.First it creates a view in the fragment,
onCreateView
and then it overrides the onViewCreated
function which defines the
listener for the button to return to the first fragment.1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 if (!Util.isInternetConnected(this)) {
5 Toast.makeText(this, "You must be connected to internet!",
6 Toast.LENGTH_LONG).show()
7 }
8}
MainActivity
overrides the onCreate()
function which checks that
internet access is available, and loads the mapsetContentView(R.layout.activity_main)
as defined in
res/layout/activity_main.xml