How it works
You can open the MainActivity.kt file to see how to send
a social report from within an app while displaying an interactive map.
In this example, the social report is sent automatically when the app
is started, so long as a real or mock device position, such as GPS,
is available.
1override fun onCreate(savedInstanceState: Bundle?)
2{
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5 gemSurfaceView = findViewById(R.id.gem_surface)
6 statusText = findViewById(R.id.status_text)
7 SdkSettings.onMapDataReady = { isReady ->
8 if (isReady)
9 {
10 // Defines an action that should be done
11 // when the world map is ready (Updated/ loaded).
12 SdkCall.execute {
13 gemSurfaceView.mapView?.followPosition()
14 waitForNextImprovedPosition {
15 submitReport()
16 }
17 }
18 }
19 }
20 SdkSettings.onApiTokenRejected = {
21 showDialog("TOKEN REJECTED")
22 }
23 requestPermissions(this)
24 if (!Util.isInternetConnected(this))
25 {
26 showDialog("You must be connected to the internet!")
27 }
28}
The onCreate()
function is overridden in the
MainActivity: AppCompatActivity()
class, and checks if
internet access is available, showing a dialog message if not.
findViewById()
is used to obtain pointers to the various
graphical user interface elements where text or graphical data
is to be displayed.
When the map is loaded,
SdkSettings.onMapDataReady = {
follow position is activated so the camera follows the
position of the device (in case it is in a car in motion,
for example):
gemSurfaceView.mapView?.followPosition()
A function is called to wait until the first good quality
position of the device is received from the position sensor,
such as GPS:
waitForNextImprovedPosition {
When a good position has been obtained, the social report
is sent automatically:
submitReport()
1private fun waitForNextImprovedPosition(onEvent: (() -> Unit))
2{
3 positionListener = PositionListener {
4 if (it.isValid())
5 {
6 Util.postOnMain { showStatusMessage("On valid position") }
7 onEvent()
8 PositionService.removeListener(positionListener)
9 }
10 }
11 PositionService.addListener(positionListener, EDataType.ImprovedPosition)
12 // listen for first valid position to start the nav
13 Util.postOnMain { showStatusMessage("Waiting for valid position", true) }
14}
The PositionListener
instance is created and added to the
PositionService.addListener()
to be called when a good position
EDataType.ImprovedPosition
is received from the GPS sensor. When that happens, the function passed in
is called automatically, that is, the onEvent()
parameter, which is
submitReport()
in this case, causing the social report to be sent upon reading the first
valid device position from the sensor.
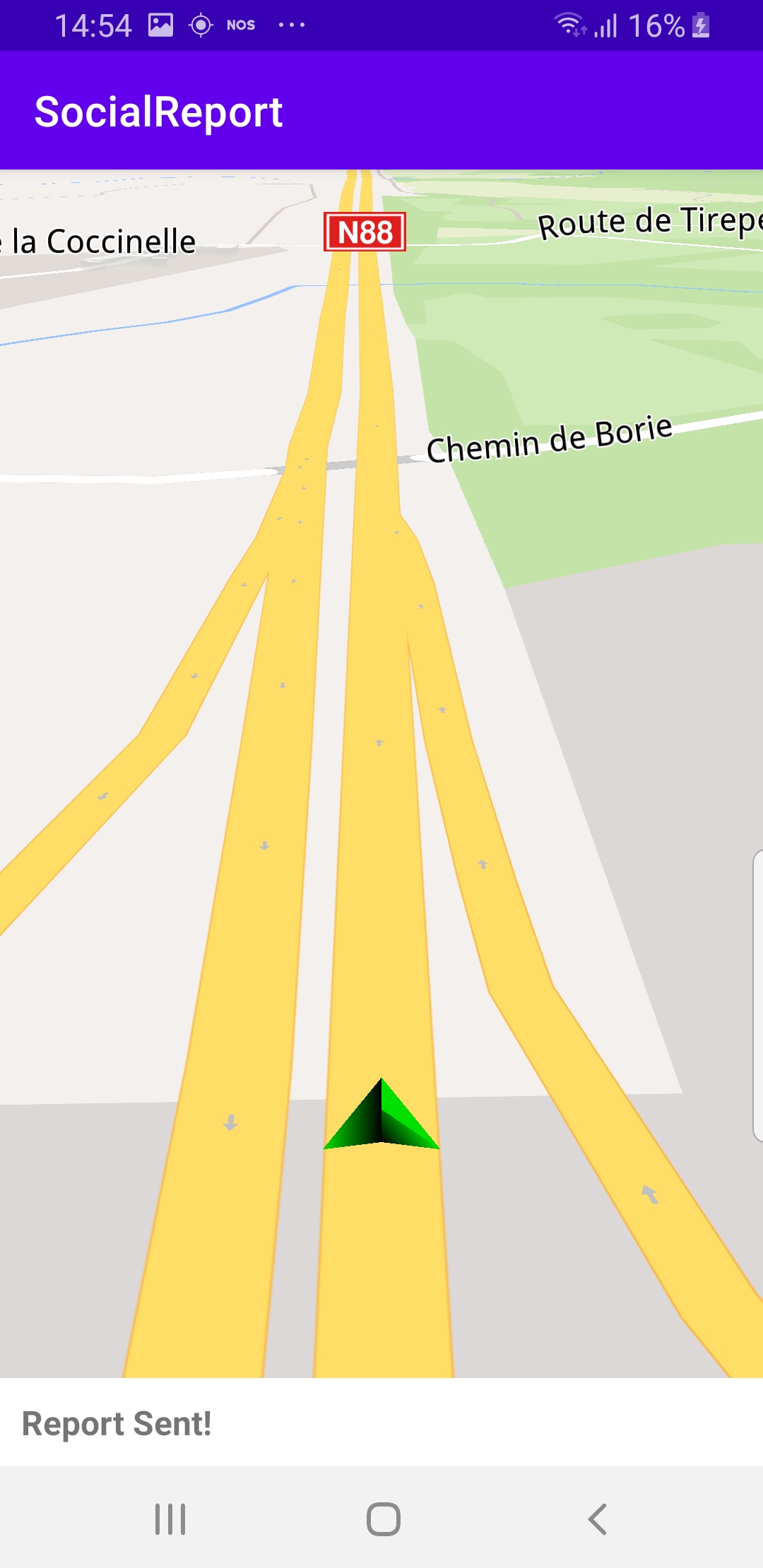
|
1private fun submitReport() = SdkCall.execute
2{
3 val overlayInfo = SocialOverlay.reportsOverlayInfo ?: return@execute
4 val countryISOCode = MapDetails().isoCodeForCurrentPosition ?: return@execute
5 val categories = overlayInfo.getCategories(countryISOCode) ?: return@execute
6 val mainCategory = categories[0] // Police
7 val subcategories = mainCategory.subcategories ?: return@execute
8 val subCategory = subcategories[0] // My side
9 val prepareIdOrError = SocialOverlay.prepareReporting()
10 if (prepareIdOrError <= 0)
11 {
12 val errorMsg = if (prepareIdOrError == GemError.NotFound
13 || prepareIdOrError == GemError.Required)
14 {
15 "Prepare error: ${GemUtil.getUIString(EStringIds.eStrGPSAccuracyIsNotGoodEnough)}"
16 }
17 else
18 {
19 "Prepare error: ${GemError.getMessage(prepareIdOrError)}"
20 }
21 Util.postOnMain { showDialog(errorMsg) }
22 return@execute
23 }
24 val error = SocialOverlay.report(prepareIdOrError, subCategory.uid, socialReportListener)
25 if (GemError.isError(error))
26 {
27 Util.postOnMain { showDialog("Report Error: ${GemError.getMessage(error)}") }
28 }
29 else
30 {
31 Util.postOnMain { showStatusMessage("Report Sent!") }
32 }
33}
The submitReport()
function creates the report:
val prepareIdOrError = SocialOverlay.prepareReporting()
and if this fails, an error message is displayed in a dialog box.
Otherwise, the report, stored in prepareIdOrError
is sent:
val error = SocialOverlay.report(prepareIdOrError, subCategory.uid, socialReportListener)
If sending fails, a dialog box is displayed, otherwise,
a status message indicating that the report was sent is displayed
at the bottom of the viewport.
Social Report¶
Setup¶
Download the
SocialReport project
archive file or clone the project with GitSee the Configure Android Example guide.
Run the example¶
In Android Studio, from the
File
menu, selectSync Project with Gradle Files
How it works¶
onCreate()
function is overridden in theMainActivity: AppCompatActivity()
class, and checks if internet access is available, showing a dialog message if not.findViewById()
is used to obtain pointers to the various graphical user interface elements where text or graphical data is to be displayed.SdkSettings.onMapDataReady = {
gemSurfaceView.mapView?.followPosition()
waitForNextImprovedPosition {
submitReport()
PositionListener
instance is created and added to thePositionService.addListener()
EDataType.ImprovedPosition
onEvent()
parameter, which issubmitReport()
submitReport()
function creates the report:val prepareIdOrError = SocialOverlay.prepareReporting()
prepareIdOrError
is sent:val error = SocialOverlay.report(prepareIdOrError, subCategory.uid, socialReportListener)
Android Examples¶
Maps SDK for Android Examples can be downloaded or cloned with Git