Public Transit Routing¶
In this guide you will learn how to render an interactive map, compute and render a public transit route on the map, and fly to the route.
Setup¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive fileDownload the PublicTransitRoutingOnMap project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
How it works¶
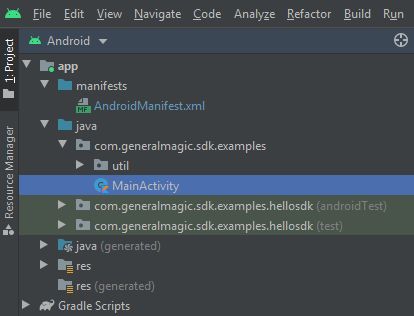
You can open the MainActivity.kt file to see how the route is computed and rendered on the map.
1// Kotlin code
2private fun calculateRoute() {
3 val waypoints = arrayListOf(
4 Landmark("San Francisco", Coordinates(37.77903, -122.41991)),
5 Landmark("San Jose", Coordinates(37.33619, -121.89058))
6 )
7
8 when (val gemError = SdkError.fromInt(reason)) {
9 SdkError.NoError -> {
10 SdkCall.execute {
11 // Get the main route from the ones that were found.
12 val mainRoute: Route? = if (routes.size > 0) {
13 routes[0]
14 } else {
15 null
16 }
17 // Add routes to the map.
18 addRoutes(routes)
19
20 if (mainRoute != null) {
21 flyToRoute(mainRoute)
22 }
23 }
24 }
25 }
26 }
27 routingService.preferences.setTransportMode(ERouteTransportMode.Public)
28 routingService.calculateRoute(waypoints)
29}
30
31private fun addRoutes(routes: ArrayList<Route>) {
32 for (routeIndex in 0 until routes.size) {
33 val route = routes[routeIndex]
34
35 // Add the route to the map so it can be displayed.
36 mapView?.preferences()?.routes()?.add(route, routeIndex == 0)
37 }
38}
39
40private fun flyToRoute(route: Route) {
41 val animation = Animation()
42 animation.setType(EAnimation.Fly)
43
44 // Center the map on a specific route using the provided animation.
45 mapView?.centerOnRoute(route, Rect(), animation)
46}
In the calculateRoute()
function, 2 Landmark
instances are
defined, one for the departure, and one for the destination
coordinates of the route endpoints. A route must have at least 2
Landmark instances(waypoints), but optionally can have more,
for any optional additional waypoints along the route.
Next, public transport mode is selected in the routing service:
routingService.preferences.setTransportMode(ERouteTransportMode.Public)
Next, the routing service computes the route, and calls the
routingService.onCompleted
callback with the resulting routes,
as there could be more than one:
routingService.calculateRoute(waypoints)
Then, addRoutes(routes)
adds the routes to the map so they are
rendered.
If there is at least one route, flyToRoute(mainRoute)
calls
the centerOnRoute()
API function to fly to the selected route,
such that its bounding box fits in the viewport.