Lane Instructions Simulated Navigation¶
In this guide you will learn how to simulate navigation
along a computed route rendered on an interactive map,
from a departure position to a desired destination.
The map is fully 3D, supporting pan, pinch-zoom,
rotate and tilt.
The navigation includes lane instruction icons which
appear at the bottom of the viewport whenever nearing
an upcoming bifurcation, or branching, of the road.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the LaneInstructions project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
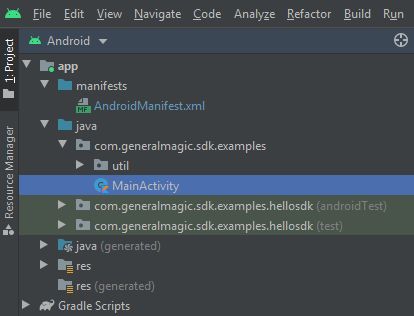
You can open the MainActivity.kt file to see how simulated navigation along a computed route with lane instruction icons works.
1private val navigationService = NavigationService()
A NavigationService()
is instantiated, which carries out
both simulated navigation and real navigation.
1private val navigationListener: NavigationListener = NavigationListener.create(
2 onNavigationStarted = {
3 SdkCall.execute {
4 gemSurfaceView.mapView?.let { mapView ->
5 mapView.preferences?.enableCursor = false
6 navRoute?.let { route ->
7 mapView.presentRoute(route)
8 }
9 enableGPSButton()
10 mapView.followPosition()
11 }
12 }
13 },
14 onDestinationReached = {
15 SdkCall.execute { gemSurfaceView.mapView?.hideRoutes() }
16 },
17 onNavigationInstructionUpdated = { instr ->
18 // Fetch the bitmap for recommended lanes.
19 val lanes = SdkCall.execute {
20 instr.laneImage?.asBitmap(150, 30, activeColor = Rgba.white())
21 }?.second
22 // Show the lanes instruction.
23 if (lanes != null) {
24 laneImage.visibility = View.VISIBLE
25 laneImage.setImageBitmap(lanes)
26 } else {
27 laneImage.visibility = View.GONE
28 }
29 }
30)
The
NavigationListener
receives event updates during navigation such as
when the destination is reached, or when the route to the desired destination
has been recomputed, because a detour away from the original route was taken.Besides the
onNavigationStarted
and onDestinationReached
listeners,
the onNavigationInstructionUpdated
listener is also instantiated,
to receive the bitmap image with the arrows for the lane(s) to follow.1private val routingProgressListener = ProgressListener.create(
2 onStarted = {
3 progressBar.visibility = View.VISIBLE
4 },
5 onCompleted = { _, _ ->
6 progressBar.visibility = View.GONE
7 },
8 postOnMain = true
9)
Define a listener to indicate when the route computation is completed,
so real or simulated navigation (simulated in this case) can start.
When navigation is started,
mapView.followPosition()
causes
the camera to follow the green arrow.This is called in the
onNavigationStarted
listener above,
right after enableGPSButton()
.If the user pans (moves) the map to another location,
the camera no longer follows the green arrow.
1private fun enableGPSButton() {
2 // Set actions for entering/ exiting following position mode.
3 gemSurfaceView.mapView?.apply {
4 onExitFollowingPosition = {
5 followCursorButton.visibility = View.VISIBLE
6 }
7 onEnterFollowingPosition = {
8 followCursorButton.visibility = View.GONE
9 }
10 // Set on click action for the GPS button.
11 followCursorButton.setOnClickListener {
12 SdkCall.execute { followPosition() }
13 }
14 }
15}
enableGPSButton()
causes a round purple button to appear in the lower
right corner of the screen, whenever the simulation is active and
the camera is not following the green arrow. If the user pushes this button,
the followPosition()
function is called, and thus the camera
starts to follow the green arrow once again.1private fun startSimulation() = SdkCall.execute {
2 val waypoints = arrayListOf(
3 Landmark("Start", 48.56785, 7.73205),
4 Landmark("Destination", 48.68695, 7.72911)
5 )
6 navigationService.startSimulation(waypoints, navigationListener, routingProgressListener)
7}
The starting, or departure point of the route is the first waypoint in a list of
2 or more Landmarks (2 in this case), each containing a name, latitude (in degrees)
and longitude (in degrees). The destination point is the last waypoint in the list.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 progressBar = findViewById(R.id.progressBar)
5 gemSurfaceView = findViewById(R.id.gem_surface)
6 laneImage = findViewById(R.id.laneImage)
7 followCursorButton = findViewById(R.id.followCursor)
8 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
9 if (!isReady) return@onMapDataReady
10 // Defines an action that should be done when
11 // the world map is ready (updated / loaded).
12 startSimulation()
13 }
14 SdkSettings.onApiTokenRejected = {
15 Toast.makeText(this@MainActivity, "TOKEN REJECTED",
16 Toast.LENGTH_SHORT).show()
17 }
18 if (!Util.isInternetConnected(this)) {
19 Toast.makeText(this, "You must be connected to internet!",
20 Toast.LENGTH_LONG).show()
21 }
22}
The
MainActivity
overrides the onCreate()
function which
checks that an internet connection is available and then starts the
simulation when the map is ready (loaded / instantiated).startSimulation()
During simulated navigation, the
onNavigationInstructionUpdated
listener causes lane instruction bitmap icons with arrows showing the
recommended lane(s) to appear at the bottom of the viewport before
every upcoming branching, or bifurcation, in the road traveled.