Apply Map Style¶
In this guide you will learn how to list the available map styles in the online content store, download a map style and change the map style used to render an interactive map.
Setup¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive fileDownload the ApplyMapStyle project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
The example downloads a preset map style from the server, in this case, a map style with topography, and applies it to the map.
The map is interactive and fully 3D, supporting pan, pinch-zoom, rotate and tilt.
How it works¶
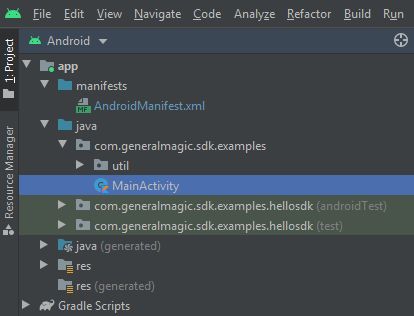
You can open the MainActivity.kt file to see how to change the map style.
1private val contentStore = ContentStore()
First, a content store item is defined, so that map styles can be requested from it.
1private fun fetchAvailableStyles() = SdkCall.execute {
2 // Call to the content store to asynchronously retrieve the list of map styles.
3 contentStore.asyncGetStoreContentList(EContentType.ViewStyleHighRes,
4 onStarted = {
5 progressBar.visibility = View.VISIBLE
6 showStatusMessage("Started content store service.")
7 },
8 onCompleted = onCompleted@{ styles, errorCode, _ ->
9 progressBar.visibility = View.GONE
10 showStatusMessage("Content store service completed, error code: $errorCode")
11 when (errorCode) {
12 GemError.NoError -> {
13 if (styles.size == 0) return@onCompleted
14 // The map style items list is not empty
15 // or null so we will select an item.
16 var style = styles[0]
17 if (styles.size > 1) // does it have a middle element?
18 style = styles[(styles.size / 2) - 1]
19 showStatusMessage("Preparing download.", true)
20 startDownloadingStyle(style)
21 }
22 GemError.Cancel -> {
23 // The action was cancelled.
24 return@onCompleted
25 }
26 else -> {
27 // There was a problem at retrieving the content store items.
28 showDialog("Content store service error: ${GemError.getMessage(errorCode)}")
29 }
30 }
31 })
32}
contentStore.asyncGetStoreContentList()
launches the request to get the list.
The onStarted
listener is called when the content store service is started,
and the list of styles download started.onCompleted
listener is defined to detect when the list of styles download
is complete. 1private fun startDownloadingStyle(style: ContentStoreItem) = SdkCall.execute {
2 val styleName = style.name ?: "NO_NAME"
3 // Start downloading a map style item.
4 style.asyncDownload(
5 onStarted = {
6 showStatusMessage("Started downloading $styleName.")
7 onDownloadStarted(style)
8 },
9 onStatusChanged = { status ->
10 onStatusChanged(status)
11 },
12 onProgress = { progress ->
13 onProgressUpdated(progress)
14 },
15 onCompleted = { _, _ ->
16 showStatusMessage("$styleName was downloaded. Applying style...")
17 applyStyle(style)
18 showStatusMessage("Style $styleName was applied.")
19 styleContainer.visibility = View.GONE
20 }
21 )
22}
This is the function that the above listener calls to download a style from the content store. Note that the function automatically applies the new map style as soon as the download is complete.
1private fun applyStyle(style: ContentStoreItem) = SdkCall.execute {
2 // Apply the style to the main map view.
3 gemSurfaceView.mapView?.preferences?.setMapStyleById(style.id)
4}
Set the map to use the newly downloaded map style.
1override fun onCreate(savedInstanceState: Bundle?)
2{
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5 progressBar = findViewById(R.id.progress_bar)
6 gemSurfaceView = findViewById(R.id.gem_surface)
7 statusText = findViewById(R.id.status_text)
8 statusProgressBar = findViewById(R.id.status_progress_bar)
9 styleContainer = findViewById(R.id.style_container)
10 styleName = findViewById(R.id.style_name)
11 downloadProgressBar = findViewById(R.id.download_progress_bar)
12 downloadedIcon = findViewById(R.id.downloaded_icon)
13 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
14 if (!isReady) return@onMapDataReady
15 fetchAvailableStyles()
16 }
17 SdkSettings.onApiTokenRejected = {
18 showDialog("TOKEN REJECTED")
19 }
20 if (!Util.isInternetConnected(this))
21 {
22 showDialog("You must be connected to internet!")
23 }
24}
MainActivity
overrides the onCreate()
function which checks that internet
access is available, and then calls the fetchAvailableStyles()
function shown
above to get the list of map styles from the content store on the server.