Free Text Search¶
In this guide you will learn how to search on
an interactive map.
Setup¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive fileDownload the Search project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
In the search bar at the top, start typing the name of
a point of interest, such as a town, airport, etc, and
text results will start to appear below as you type.
How it works¶
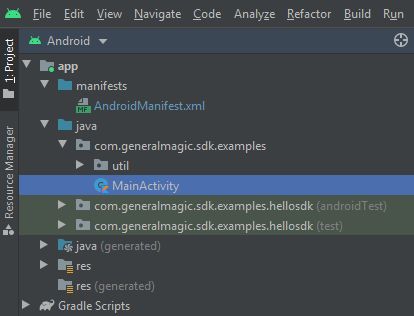
You can open the MainActivity.kt file to see how the interactive map is rendered.
1class MainActivity : AppCompatActivity()
2{
3 private var searchService = SearchService()
In MainActivity
a SearchService()
instance is obtained.
1searchInput.setOnQueryTextListener(
2 object : SearchView.OnQueryTextListener
3 {
4 override fun onQueryTextSubmit(query: String?): Boolean
5 {
6 searchInput.clearFocus()
7 return true
8 }
9 override fun onQueryTextChange(newText: String?): Boolean
10 {
11 applyFilter(newText ?: "")
12 return true
13 }
14 }
15)
If the user input text to search changes or is submitted, the text is set
in the filter to use for searching by the applyFilter()
function.
1private fun search(filter: String): Int
2{
3 return SdkCall.execute
4 {
5 // Get the current position of the user.
6 val position = PositionService().getPosition()
7 val latitude = position?.getLatitude() ?: 0.0
8 val longitude = position?.getLongitude() ?: 0.0
9 val coordinates = Coordinates(latitude, longitude)
10 searchService.preferences.setSearchAddresses(true)
11 searchService.preferences.setSearchMapPOIs(true)
12 searchService.searchByFilter(filter, coordinates) onCompleted@{ results, reason, _ ->
13 val gemError = SdkError.fromInt(reason)
14 if (gemError == SdkError.Cancel) return@onCompleted
15 val adapter = CustomAdapter(results)
16 listView?.adapter = adapter
17 progressBar?.visibility = View.GONE
18 if (reason == SdkError.NoError.value && results.isEmpty())
19 {
20 Toast.makeText(
21 this@MainActivity, "No results!", Toast.LENGTH_SHORT
22 ).show()
23 }
24 }
25 } ?: SdkError.Cancel.value
26}
The
search()
function uses the SearchService()
instance to first
set preferences to search for both addresses and POIs (points of interest)searchService.preferences.setSearchAddresses(true)
searchService.preferences.setSearchMapPOIs(true)
and then it starts the search with
searchByFilter
and also defines
an onCompleted
callback which receives the results:searchService.searchByFilter(filter, coordinates) onCompleted@{