Route Terrain Profile¶
In this guide you will learn how to obtain and
display terrain statistics for a route, given the
coordinates of the departure and destination points.
The terrain profile statistics include the minimum
and the maximum elevation above sea level in meters,
as well as the total vertical meters climbed,
and the total vertical meters descended during
the route.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the RouteTerrainProfile project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
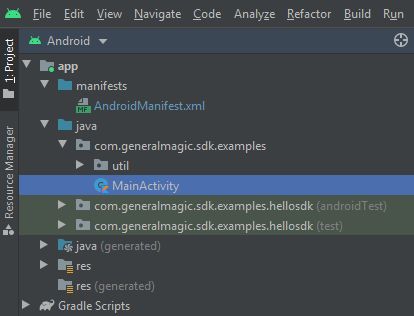
You can open the MainActivity.kt file to see how the route terrain profile statistics are obtained and displayed.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 progressBar = findViewById(R.id.progressBar)
5
6 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
7 if (!isReady) return@onMapDataReady
8 // Defines an action that should be done after the world map is ready.
9 calculateRoute()
10 }
11 SdkSettings.onApiTokenRejected = {
12 Toast.makeText(this, "TOKEN REJECTED", Toast.LENGTH_LONG).show()
13 }
14 if (!GemSdk.initSdkWithDefaults(this)) {
15 // The SDK initialization was not completed.
16 finish()
17 }
18 if (!Util.isInternetConnected(this)) {
19 Toast.makeText(this, "You must be connected to internet!", Toast.LENGTH_LONG).show()
20 }
21}
The
MainActivity
overrides the onCreate
function which checks that
internet access is available using Util.isInternetConnected()
and calls
the calculateRoute()
function after the map is instantiated, as indicated
by the isReady
flag. 1private fun calculateRoute() = SdkCall.execute {
2 val waypoints = arrayListOf(
3 Landmark("Zaragoza", 41.645, -0.883),
4 Landmark("Toulouse", 43.6, 1.438)
5 )
6 /*
7 Setting setBuildTerrainProfile(true) in the Routing Service preferences
8 is mandatory to get data related to the route terrain profile,
9 otherwise the terrain profile is not calculated in the routing process.
10 */
11 routingService.preferences.buildTerrainProfile = true
12 routingService.calculateRoute(waypoints)
13}
The
calculateRoute()
function computes a route between two or more landmarks,
given as a list of waypoints, where the first one is the departure point, and
the last one is the destination point.The landmarks contain a name, latitude and longitude, in degrees.
routingService.preferences.buildTerrainProfile = true
has to be set to true
before routingService.calculateRoute(waypoints)
in order to also get the
terrain profile statistics. 1@SuppressLint("SetTextI18n")
2private fun displayTerrainInfo(terrain: RouteTerrainProfile) {
3 var maxElv = .0f
4 var minElv = .0f
5 var elevationAt = .0f
6 var totalUp = .0f
7 var totalDown = .0f
8 var climbingSections = 0
9
10 SdkCall.execute {
11 maxElv = terrain.maxElevation
12 minElv = terrain.minElevation
13 elevationAt = terrain.getElevation(1000) // 1 KM
14 totalUp = terrain.totalUp
15 totalDown = terrain.totalDown
16 climbingSections = terrain.climbSections?.size ?: 0
17 }
18 findViewById<TextView>(R.id.text).text =
19 "Details: \nMin. Elevation = $minElv m, " +
20 "\nMax. Elevation = $maxElv m, " +
21 "\nElevation after 1KM = $elevationAt m," +
22 "\nTotal Up = $totalUp m, \n" +
23 "Total Down = $totalDown m \n\n\n" +
24 "Number of Climbing Section: \n$climbingSections"
25}
The above function,
displayTerrainInfo()
displays some basic
statistics about the computed route in this example in text format,
to show how to access the terrain profile data.The RouteTerrainProfile class contains a lot of information, but this example
presents only the minimum and maximum elevation above sea level in meters,
elevation at a certain point along the route, the total vertical meters ascending
and descending, and the number of climbing sections in the selected route.
To display a chart representing the elevation of the route,
the method
getElevationSamples(samplesCount)
can be used, specifying the
number of samples, and thus resolution, of the resulting terrain data.There are also additional methods about route terrain profile in the documentation.