Routing¶
In this guide you will learn how to compute a route
from a departure point to a destination point
and display the route length in kilometers, and
duration in hours:minutes as text on screen.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the Routing project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
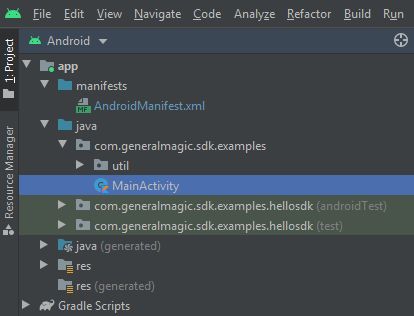
You can open the MainActivity.kt file to see how the route is computed and the statistics of the computed route are displayed.
1private val routingService = RoutingService(
2 onStarted = {
3 progressBar.visibility = View.VISIBLE
4 },
5 onCompleted = onCompleted@{ routes, errorCode, _ ->
6 progressBar.visibility = View.GONE
7 when (errorCode) {
8 GemError.NoError -> {
9 if (routes.size == 0) return@onCompleted
10 // Get the main route from the ones that were found.
11 displayRouteInfo(formatRouteName(routes[0]))
12 }
13 GemError.Cancel -> {
14 // The routing action was canceled.
15 }
16 else -> {
17 // There was a problem at computing the routing operation.
18 Toast.makeText(
19 this@MainActivity,
20 "Routing service error: ${GemError.getMessage(errorCode)}",
21 Toast.LENGTH_SHORT
22 ).show()
23 }
24 }
25 }
26)
A RoutingService()
is instantiated, to compute a route,
with an onStarted
and an onCompleted
listener, to receive
notifications from the routing service when a route computation
starts and when it is completed.
1private fun calculateRoute() = SdkCall.execute {
2 val wayPoints = arrayListOf(
3 Landmark("Frankfurt am Main", 50.11428, 8.68133),
4 Landmark("Karlsruhe", 49.0069, 8.4037),
5 Landmark("Munich", 48.1351, 11.5820)
6 )
7 routingService.calculateRoute(wayPoints)
8}
The starting, or departure point of the route is the first waypoint in a list of
2 or more Landmarks (3 in this case), each containing a name, latitude (in degrees)
and longitude (in degrees). The destination point is the last waypoint in the list.
The
calculateRoute()
function passes the list of waypoints to the routing
service to calculate the route: routingService.calculateRoute(wayPoints)
. 1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 progressBar = findViewById(R.id.progressBar)
5
6 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
7 if (!isReady) return@onMapDataReady
8 // Defines an action that should be done after the world map is updated.
9 calculateRoute()
10 }
11 SdkSettings.onApiTokenRejected = {
12 Toast.makeText(this, "TOKEN REJECTED", Toast.LENGTH_LONG).show()
13 }
14 if (!GemSdk.initSdkWithDefaults(this)) {
15 // The SDK initialization was not completed.
16 finish()
17 }
18 if (!Util.isInternetConnected(this)) {
19 Toast.makeText(this, "You must be connected to internet!",
20 Toast.LENGTH_LONG).show()
21 }
22}
The
MainActivity
overrides the onCreate()
function which checks that
internet access is available, and then, when the map is instantiated
and ready (although the map is not rendered in this example), starts the
route calculation: calculateRoute()
.1private fun displayRouteInfo(routeName: String) {
2 findViewById<TextView>(R.id.text).text = routeName
3}
The
routingService
listener above receives the onCompleted
notification
when the route computation is complete, and if there is at least one resulting
route, then it takes the first route in the list (at index 0) and calls
displayRouteInfo(formatRouteName(routes[0]))
to print the route length
in kilometers and duration in hours:minutes as text on screen.The route statistics text printed by
displayRouteInfo()
is formatted by
formatRouteName()
: 1private fun formatRouteName(route: Route): String = SdkCall.execute {
2 val timeDistance = route.timeDistance ?: return@execute ""
3 val distInMeters = timeDistance.totalDistance
4 val timeInSeconds = timeDistance.totalTime
5 val distTextPair = GemUtil.getDistText(
6 distInMeters,
7 SdkSettings.unitSystem,
8 bHighResolution = true
9 )
10 val timeTextPair = GemUtil.getTimeText(timeInSeconds)
11 var wayPointsText = ""
12 route.waypoints?.let { wayPoints ->
13 for (point in wayPoints) {
14 wayPointsText += (point.name + "\n")
15 }
16 }
17 return@execute String.format(
18 "$wayPointsText \n\n ${distTextPair.first} ${distTextPair.second} \n " +
19 "${timeTextPair.first} ${timeTextPair.second}"
20 )
21} ?: ""