Set TTS Language¶
In this guide you will learn how to set or change
the TTS (text to speech) voice instruction language.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the SetTTSLanguage project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
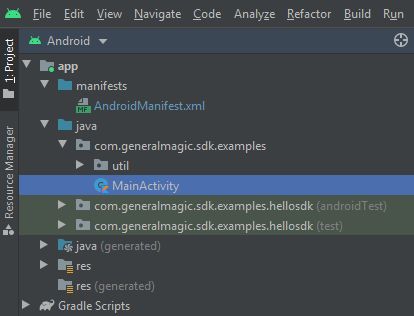
You can open the MainActivity.kt file to see how setting the TTS (text to speech) voice instruction language works.
1override fun onCreate(savedInstanceState: Bundle?)
2{
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5 progressBar = findViewById(R.id.progressBar)
6 selectedLanguageTextView = findViewById(R.id.language_value)
7 languageContainer = findViewById(R.id.language_container)
8 playButton = findViewById(R.id.play_button)
9 languageContainer.setOnClickListener {
10 onLanguageContainerClicked()
11 }
12 playButton.setOnClickListener {
13 SdkCall.execute {
14 SoundPlayingService.playText(GemUtil.getTTSString(EStringIds.eStrMindYourSpeed),
15 SoundPlayingListener(), SoundPlayingPreferences())
16 }
17 }
18 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
19 if (!isReady) return@onMapDataReady
20 if (!SoundPlayingService.ttsPlayerIsInitialized)
21 {
22 SoundUtils.addTTSPlayerInitializationListener(this)
23 }
24 else
25 {
26 loadTTSLanguages()
27 }
28 }
29 SdkSettings.onApiTokenRejected = {
30 showDialog("TOKEN REJECTED")
31 }
32 // This step of initialization is mandatory for using the SDK without a map.
33 if (!GemSdk.initSdkWithDefaults(this))
34 {
35 // The SDK initialization was not completed.
36 finish()
37 }
38 if (!Util.isInternetConnected(this))
39 {
40 showDialog("You must be connected to internet!")
41 }
42}
The
MainActivity
overrides the onCreate()
function which checks
that an internet connection is available and loads the TTS languages
loadTTSLanguages()
when the map instantiation is complete (the map is ready).
If the TTS player is not initialized, a listener is set up to wait for
the TTS player to be initialized, which causes the TTS languages to
be loaded by the callback function defined for that listener:override fun onTTSPlayerInitialized() { loadTTSLanguages() }
.A click listener is set for clicking on the name of the currently
active language
languageContainer.setOnClickListener { onLanguageContainerClicked() }
to display the list of TTS languages.A click listener is set for the play button
playButton.setOnClickListener
at the bottom of the screen to play the “mind your speed” messageSoundPlayingService.playText(GemUtil.getTTSString(EStringIds.eStrMindYourSpeed),
in the currently selected TTS language.1private fun loadTTSLanguages()
2{
3 SdkCall.execute {
4 ttsLanguages = SoundPlayingService.getTTSLanguages()
5 }
6 runOnUiThread {
7 onTTSLanguagesLoaded()
8 }
9}
The function to load the TTS languages.
1private fun onTTSLanguagesLoaded()
2{
3 selectedLanguageTextView.text = ttsLanguages[selectedLanguageIndex].name
4 SoundPlayingService.setTTSLanguage(ttsLanguages[selectedLanguageIndex].code)
5 progressBar.visibility = View.GONE
6 languageContainer.visibility = View.VISIBLE
7 playButton.visibility = View.VISIBLE
8}
When the TTS languages are loaded, the currently selected language,
initially the first one (at index 0) is displayed:
private var selectedLanguageIndex = 0
1private fun onLanguageContainerClicked()
2{
3 val builder = AlertDialog.Builder(this)
4 val convertView = layoutInflater.inflate(R.layout.dialog_list, null)
5 val listView = convertView.findViewById<RecyclerView>(R.id.list_view).apply {
6 layoutManager = LinearLayoutManager(this@MainActivity)
7 addItemDecoration(DividerItemDecoration(applicationContext, (layoutManager as LinearLayoutManager).orientation))
8 setBackgroundResource(R.color.white)
9 val lateralPadding = resources.getDimension(R.dimen.bigPadding).toInt()
10 setPadding(lateralPadding, 0, lateralPadding, 0)
11 }
12 val adapter = CustomAdapter(selectedLanguageIndex, ttsLanguages)
13 listView.adapter = adapter
14 builder.setView(convertView)
15 val dialog = builder.create()
16 dialog.show()
17 adapter.dialog = dialog
18}
When the currently selected language is clicked,
a
CustomAdapter
is used to display the list of available TTS languages.1...
2itemView.setOnClickListener {
3 selectedLanguageIndex = position
4 SoundPlayingService.setTTSLanguage(dataSet[position].code)
5 selectedLanguageTextView.text = dataSet[position].name
6 dialog?.dismiss()
7 }
8...
Inside the
CustomAdapter
, clicking on a TTS language in the list
causes that language to be set in the sound playing service:SoundPlayingService.setTTSLanguage(dataSet[position].code)
.