Hello Map¶
In this guide you will learn how to render
an interactive map centered on a desired location.
The map is fully 3D, supporting pan, pinch-zoom,
rotate and tilt.
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the HelloMap project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
How it works¶
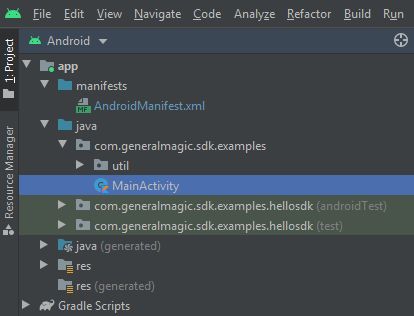
You can open the MainActivity.kt file to see how the interactive map is rendered.
1class MainActivity : AppCompatActivity() {
2 override fun onCreate(savedInstanceState: Bundle?) {
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5 if (!Util.isInternetConnected(this)) {
6 Toast.makeText(this, "You must be connected to internet!",
7 Toast.LENGTH_LONG).show()
8 }
9 }
10 override fun onDestroy() {
11 super.onDestroy()
12 // Deinitialize the SDK.
13 GemSdk.release()
14 }
15 override fun onBackPressed() {
16 finish()
17 exitProcess(0)
18 }
19}
The
MainActivity
overrides the onCreate
function which checks that
internet access is available, and instantiates and loads the map:setContentView(R.layout.activity_main)
as defined in res/layout/activity_main.xml
in the project.The
onBackPressed()
callback function exits the app when the
back button is pressed on the device.