Position Log Playback¶
In this guide you will learn how to play back
a previously recorded position (GPS) log, using DataSource
on an interactive map, displayed using MapView
,
with frame per second (FPS) counter.
GPS Log Data Source¶
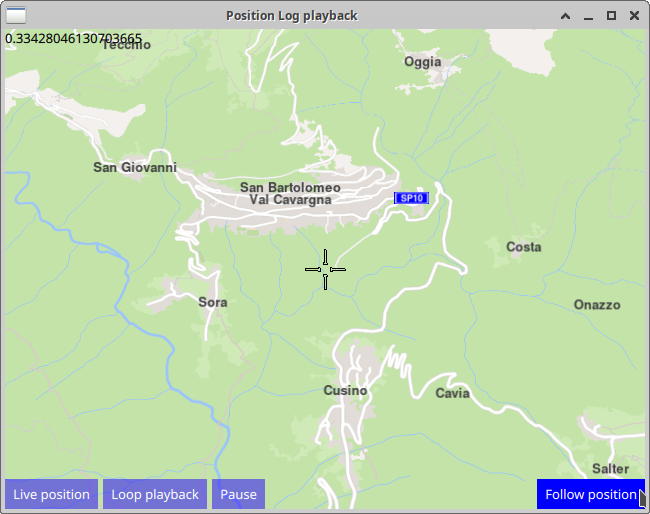
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
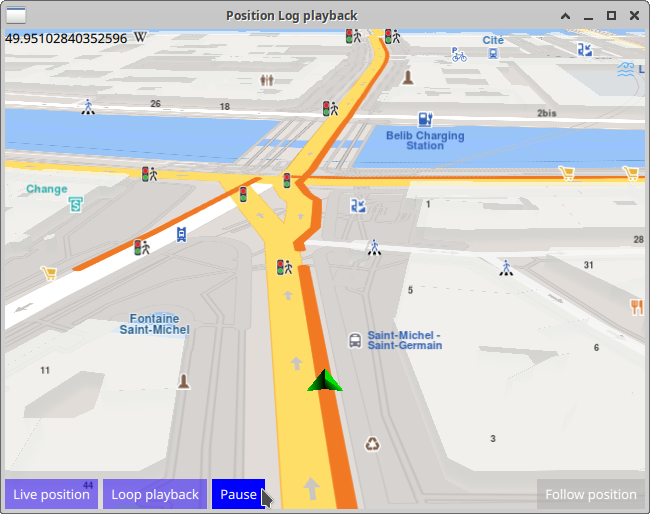
PositionLogPlayback
demonstrates how easy it is to use MapView
to display an interactive map, and play back a pre-recorded position (GPS) log, using DataSource
The input position (GPS) logfile has to be generated/saved in the .nmea
format.
Playback can be paused and resumed, and also set to repeat continuously (loop).
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the PositionLogPlayback example folder and open PositionLogPlayback.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
In main.qml, in the Component.onCompleted:
block, the datasource is
set by specifying the filename of a previously recorded [GPS] position log:
ServicesManager.dataSource.playbackFile = Qt.resolvedUrl("gpslog_paris.nmea");
Also the content type that will be downloaded upon demand is specified:
let updater = ServicesManager.contentUpdater(ContentItem.Type.RoadMap);
The MapView
displaying the interactive map also has 4 buttons,
the one on the bottom left of the viewport to start/stop the position
datasource playback, and the one on the bottom right to start/resume
following position.
Playback¶
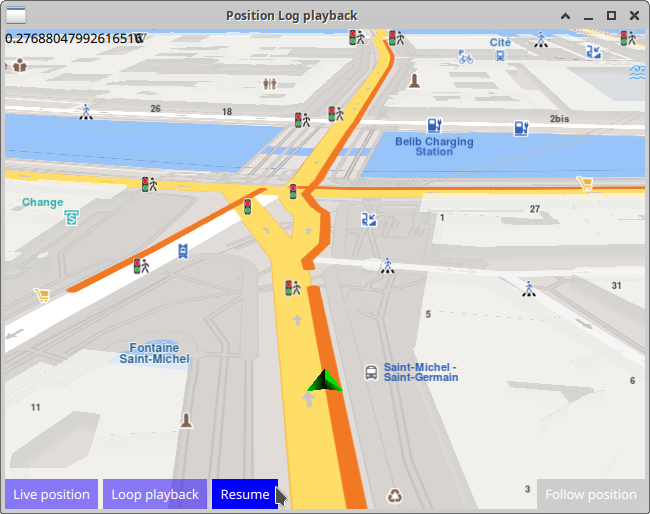
1Button {
2 text: ServicesManager.dataSource.type === DataSource.Type.Live
3 ? "Position Log playback" : "Live position"
4 background: Rectangle {
5 opacity: parent.hovered ? 1 : 0.5
6 color: enabled ? parent.down ? "#aa00aa" :
7 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
8 }
9 palette { buttonText: "#ffffff"; }
10 onClicked: ServicesManager.dataSource.type = ServicesManager.dataSource.type
11 === DataSource.Type.Live ? DataSource.Type.Playback : DataSource.Type.Live;
12}
Playback starts automatically when the data source type is set to
DataSource.Type.Playback
and stops when the data source type is set to
DataSource.Type.Live
which means that the position indicator on the
map shows the actual position of the device, obtained from the
location (GPS) sensor.
To restart playback from the beginning, click Live position
to switch to
live GPS position sensor input, then click Position Log playback
Follow Position¶
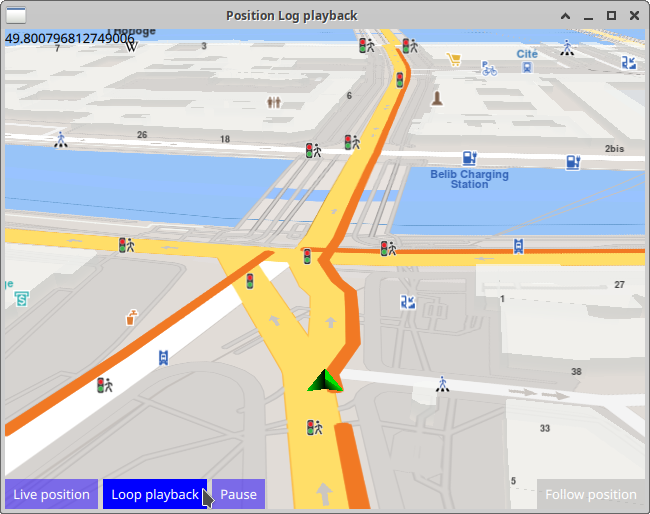
1Button {
2 anchors.right: parent.right
3 anchors.bottom: parent.bottom
4 text: qsTr("Follow position")
5 enabled: !mapView.followingPosition
6 background: Rectangle {
7 opacity: parent.hovered ? 1 : 0.5
8 color: enabled ? parent.down ? "#aa00aa" :
9 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
10 }
11 palette { buttonText: "#ffffff"; }
12 onClicked: mapView.followingPosition = true
13}
Panning the map during simulation/playback will stop follow position, and the button needs to be clicked again to resume following position.
Follow position
means that the camera flies to the position
of the green arrow and then follows the simulated position arrow.
This is necessary, as the arrow is likely to be located elsewhere
on the map, not at the current location of the camera.
Pause / Resume Playback¶
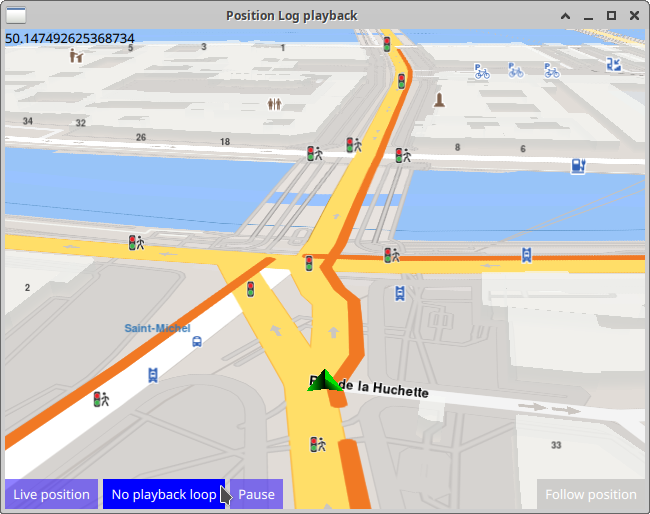
The green position arrow plays back the previously recorded positions on the map, from the data source specified above.
1Button {
2 id: pauseResume
3 text: "Pause"
4 background: Rectangle {
5 opacity: parent.hovered ? 1 : 0.5
6 color: enabled ? parent.down ? "#aa00aa" :
7 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
8 }
9 palette { buttonText: "#ffffff"; }
10 onClicked: {
11 if ( ServicesManager.dataSource.playbackStatus === DataSource.PlaybackStatus.Paused ) {
12 ServicesManager.dataSource.resume(); pauseResume.text = "Pause"
13 } else {
14 ServicesManager.dataSource.pause(); pauseResume.text = "Resume"
15 }
16 }
17}
The Pause / Resume
button pauses and continues playback of the pre-recorded
position (GPS) log file, which is in the .nmea
format,
by calling the pause()
and resume()
functions, respectively.
Continuous Playback Loop¶
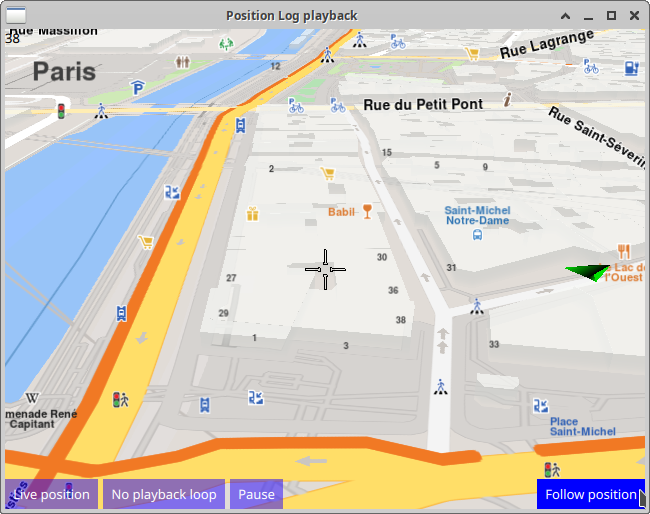
1Button {
2 text: ServicesManager.dataSource.playbackLoopMode
3 ? "No playback loop" : "Loop playback"
4 background: Rectangle {
5 opacity: parent.hovered ? 1 : 0.5
6 color: enabled ? parent.down ? "#aa00aa" :
7 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
8 }
9 palette { buttonText: "#ffffff"; }
10 onClicked: ServicesManager.dataSource.playbackLoopMode =
11 !ServicesManager.dataSource.playbackLoopMode;
12}
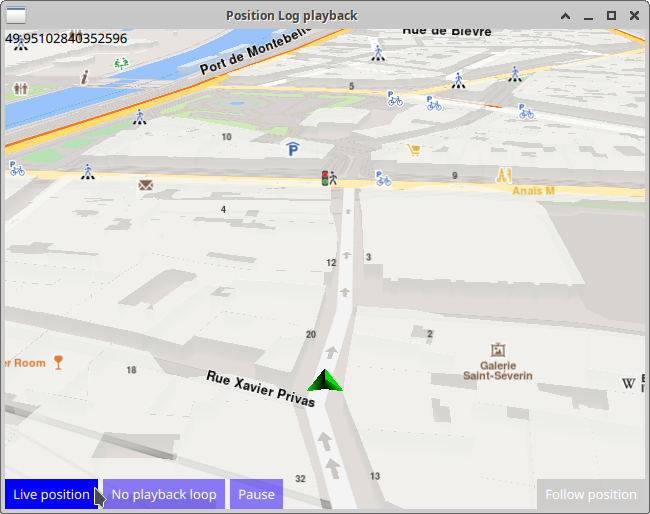
The Loop playback
button toggles the e playbackLoopMode true/false flag;
when this flag is true, playback is continuous, starting again from the
beginning after completing the playback of the position (GPS) log file.