Map Perspective Change¶
In this guide you will learn how to automatically
tilt the map to switch between 3D/perspective view and
2D view(looking vertically downward).
Setup¶
First, get an API key token, see the
Getting Started guide.
Download the MapPerspectiveChange project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example¶
In Android Studio, from the File
menu, select Sync Project with Gradle Files
An android device should be connected via USB cable.
Press SHIFT+F10 to compile, install and run the example on the
android device.
The map on the left is in 2D mode, looking vertically downward.
Pressing the button will switch the map to 3D mode.
The map on the right is in perspective/3D mode, and pressing the
button will switch the map back to 2D mode.
How it works¶
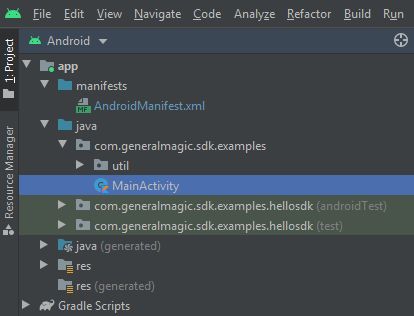
You can open the MainActivity.kt file to see how the map is tilted automatically between 2D(looking vertically downward) and 3D modes.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 surfaceView = findViewById(R.id.gem_surface)
5 button = findViewById(R.id.button)
6 val twoDimensionalText = resources.getString(R.string.two_dimensional)
7 val threeDimensionalText = resources.getString(R.string.three_dimensional)
8
9 button.setOnClickListener {
10 // Get the map view.
11 surfaceView.mapView?.let { mapView ->
12 // Establish the current map view perspective.
13 currentPerspective = if (currentPerspective == EMapViewPerspective.TwoDimensional) {
14 button.text = twoDimensionalText
15 EMapViewPerspective.ThreeDimensional
16 } else {
17 button.text = threeDimensionalText
18 EMapViewPerspective.TwoDimensional
19 }
20 SdkCall.execute {
21 // Change the map view perspective.
22 mapView.preferences?.setMapViewPerspective(
23 currentPerspective,
24 Animation(EAnimation.Linear, 300)
25 )
26 }
27 }
28 }
29 if (!Util.isInternetConnected(this)) {
30 Toast.makeText(this, "You must be connected to internet!", Toast.LENGTH_LONG).show()
31 }
32 }
The
MainActivity
overrides the onCreate
function, which checks that
internet access is available, and defines a button to toggle between 2D (vertical)
and 3D (perspective/tilted) map modes.Initially, the map is in 2D mode,
EMapViewPerspective.TwoDimensional
,
and the button displays 3D, corresponding to EMapViewPerspective.ThreeDimensional
,
the mode to switch to, when the user presses the button.After the user presses the button, the map is in 3D mode and the button displays 2D.