Markers¶
In this guide you will learn how to add an icon with an image of your choice at a coordinate of your choice on the map; also how to add a line (polyline), or a collection/set of polylines, or a polygon, or a collection of polygons, to the interactive map.
Custom icons and polygons¶
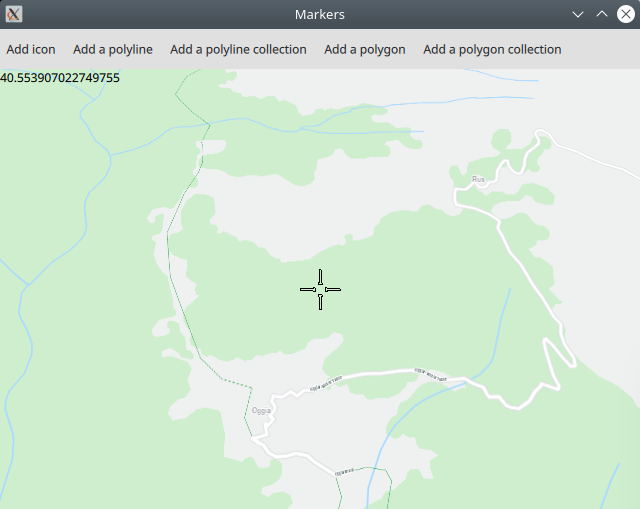
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
Markers
demonstrates how easy it is to use MapView
to display an interactive map, and add various icons, polylines
and polygons to the map.
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the Markers example folder and open Markers.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
Icons with custom image¶
In main.qml, in the "Add icon"
ToolButton block, the image to
be used on the icon is specified as follows:
ServicesManager.createIconFromFile("qrc:/kdab.png")
kdab.png
is an image file in the directory of this example.
The image filename is specified like this in the
qml.qrc
file, so that the above file URL can find it:
<file>kdab.png</file>
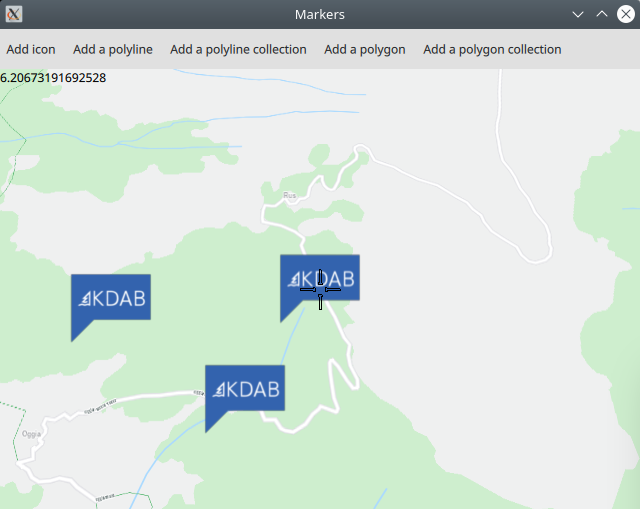
Click the "Add icon"
button in the top toolbar,
then pan or zoom the map, and then click again,
to add to the map as many icons as you like.
Polylines¶
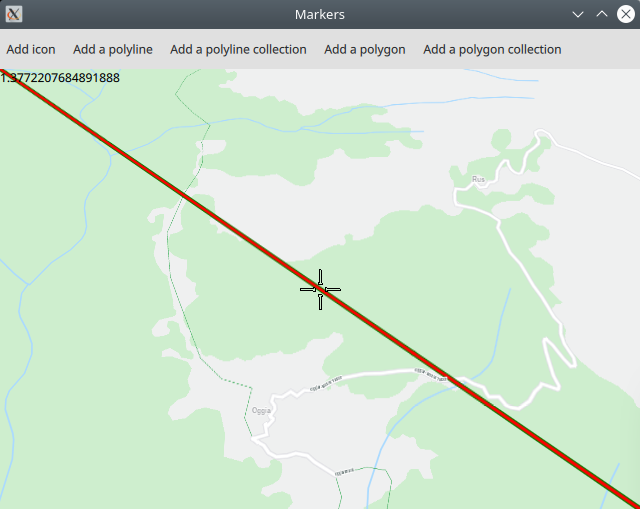
1let p1 = map.wgsForScreen(Qt.point(0, 0));
2let p2 = map.wgsForScreen(Qt.point(map.width, map.height));
In the "Add a polyline"
ToolButton block, the top left corner
of the map viewport, (0, 0)
and the bottom right corner,
(map.width, map.height)
, are converted to longitude, latitude
coordinates on the surface of the Earth, using wgsForScreen()
,
so that a polyline can be rendered on the world map between
the coordinates of the two viewport corners mentioned,
which are in view.
1let marker = ServicesManager.createMarker();
2marker.append(p1);
3marker.append(p2);
A marker is created and the two defined points are added.
1let renderSettings = ServicesManager.createMarkerRenderSettings();
2renderSettings.polylineInnerColor = "red";
3renderSettings.polylineOuterColor = "green";
4renderSettings.polylineInnerSizeMM = 3;
5renderSettings.polylineOuterSizeMM = 1;
The colors of the polyline are selected and stored in a render settings object.
1let list = map.markerCollection.getExtendedList(MarkerList.Type.Polyline);
2list.append(marker, renderSettings);
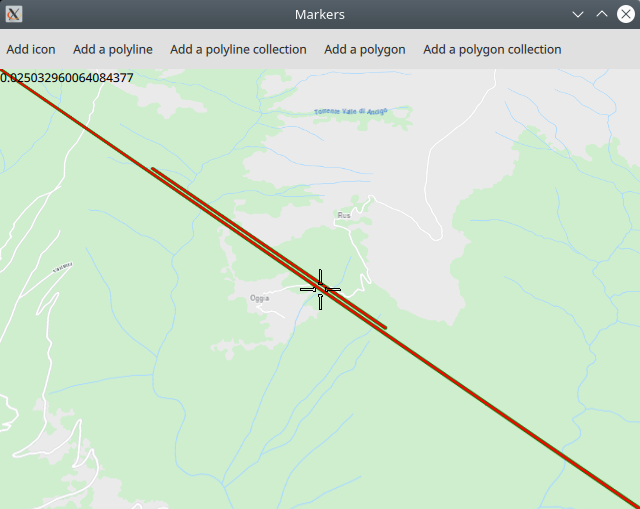
Finally, the markers are added to the map,
1MapView {
2 id: map
3 ...
4}
where map
is the id of the MapView
which renders the interactive map.
Click the "Add a polyline"
button in the top toolbar,
then pan or zoom the map, and then click again,
to add to the map as many polylines as you like.
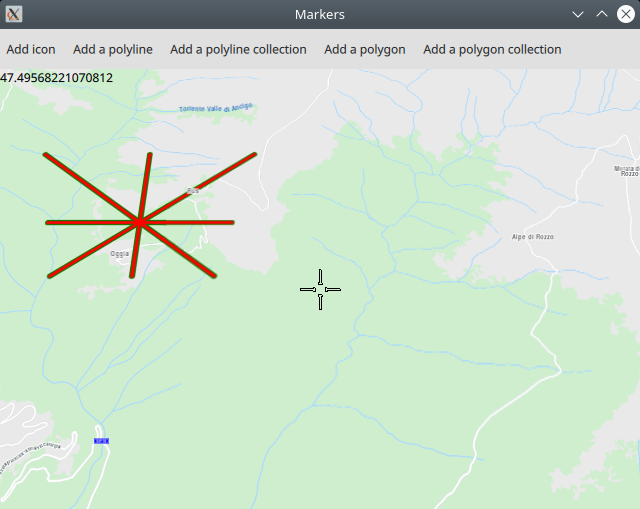
To add multiple polylines at once to the map,
let list = ServicesManager.createMarkerList(MarkerList.Type.Polyline);
a marker list can be created. Then, each polyline is added to the list as it is created:
1{
2 let marker = ServicesManager.createMarker();
3 marker.append(map.wgsForScreen(Qt.point(map.width, 0))); // top right
4 marker.append(map.wgsForScreen(Qt.point(0, map.height))); // bottom left
5 list.append(marker);
6}
In this code, a polyline is created, crossing the viewport diagonally from the top right to the bottom left, using the coordinates on the surface of the Earth which are currently in those two corners of the viewport. Thus, the polyline is actually on the surface of the Earth.
Finally,
map.markerCollection.appendList(list, renderSettings);
the entire list of markers, as well as the renderSettings object describing how the polylines are to be rendered, including color attributes, is added to the map.
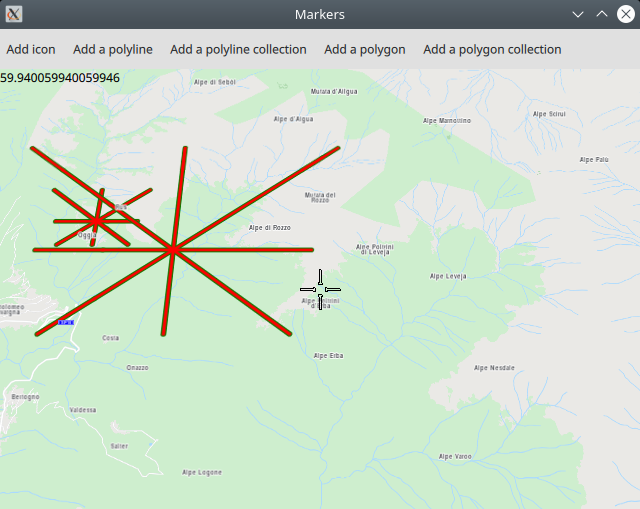
After panning or zooming the map, and then clicking the
"Add a polyline collection"
button again,
a new collection of polylines is created, using
the coordinates on the surface of the Earth which
are currently at the corners and edges of the viewport,
and rendered on the map.
Polygons¶
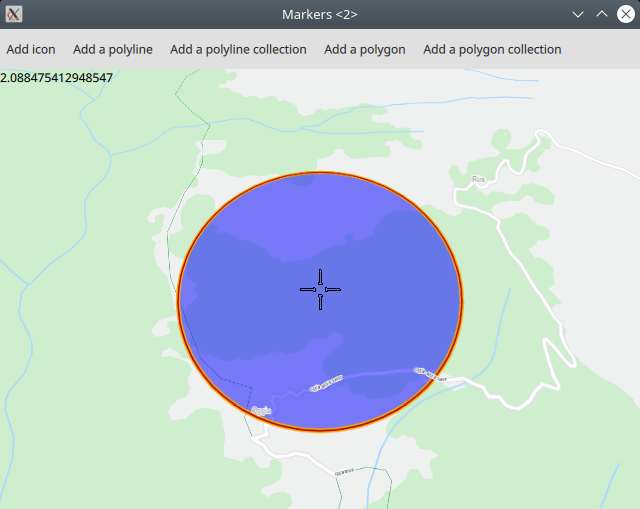
1let center = map.wgsForScreen(Qt.point(map.width / 2, map.height / 2));
2let radius = center.distance(map.wgsForScreen(Qt.point(map.width / 3, map.height / 3)));
3let marker = ServicesManager.createCirclePolygonMarker(center, radius);
In the "Add a polygon"
ToolButton block, a circle is defined,
by getting the longitude, latitude coordinates of the point on
the surface of the Earth at the viewport center, with a radius
1/3 of the viewport width and height.
1let renderSettings = ServicesManager.createMarkerRenderSettings();
2renderSettings.polygonFillColor = Qt.rgba(0, 0, 1, 0.5);
3renderSettings.polylineOuterColor = "orange";
4renderSettings.polylineOuterSizeMM = 2;
The colors for rendering the circle/polygon are set in a render settings object.
1let list = map.markerCollection.getExtendedList(MarkerList.Type.Polygon);
2list.append(marker, renderSettings);
The circle/polygon is added to the map, along with the settings specifying the colors in which it is to be rendered.
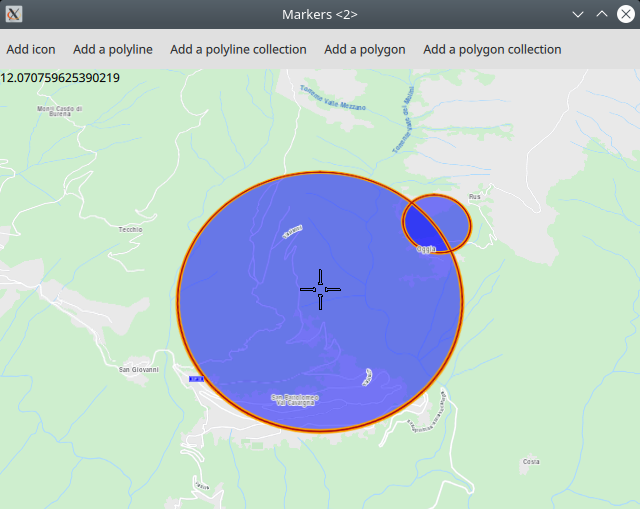
After panning or zooming the map, and then clicking the
"Add a polygon"
button again, a new circle/polygon
is created, using the coordinates on the surface of the Earth which
are currently at the viewport center, and rendered on the map.
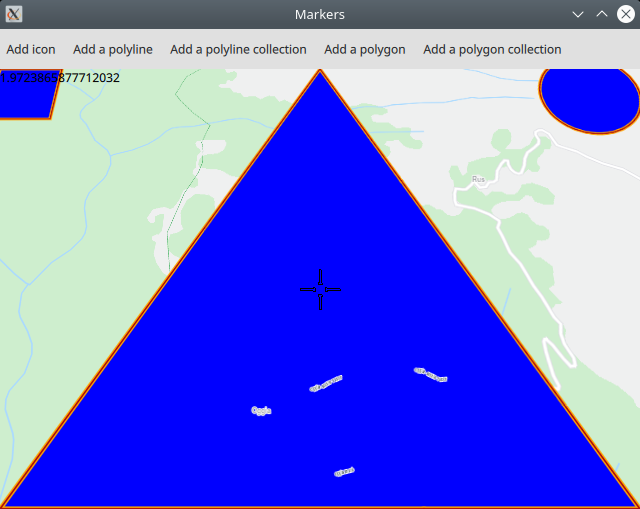
To add multiple polygons at once to the map,
let list = ServicesManager.createMarkerList(MarkerList.Type.Polygon);
a marker list can be created. Then, each polygon is added to the list as it is created:
1{
2 // create a triangle
3 let marker = ServicesManager.createMarker();
4 marker.append(map.wgsForScreen(Qt.point(map.width / 2, 0)));
5 marker.append(map.wgsForScreen(Qt.point(0, map.height)));
6 marker.append(map.wgsForScreen(Qt.point(map.width , map.height)));
7 list.append(marker);
8}
In this code, a triangle/polygon is created, between the center top of the viewport, the bottom left and the bottom right, using the coordinates on the surface of the Earth which are currently in those three points on the viewport edge. Thus, the polygon is actually on the surface of the Earth.
Finally,
map.markerCollection.appendList(list, renderSettings);
the entire list of markers, as well as the renderSettings object describing how the polygons are to be rendered, including color attributes, is added to the map.
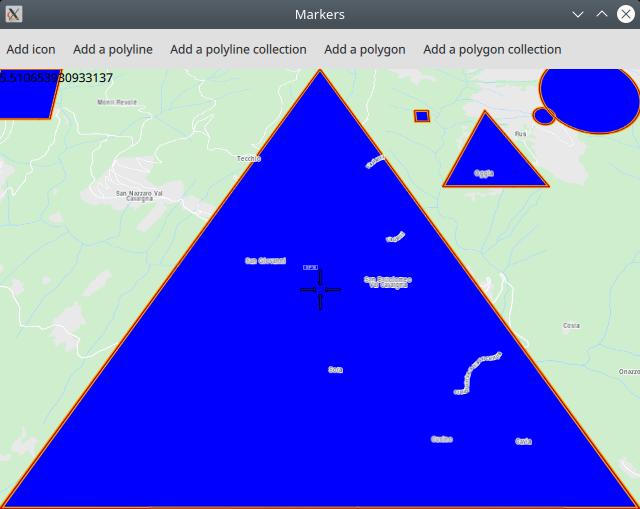
After panning or zooming the map, and then clicking the
"Add a polygon collection"
button again,
a new collection of polygons is created, using
the coordinates on the surface of the Earth which
are currently at the bottom corners and top center
edge of the viewport, and rendered on the map.