Map Coverage MultiPoint¶
In this guide you will learn how to check map coverage for a single lon,lat coordinate point, or a list of lon,lat coordinate points.
Check if map tiles are on the device¶
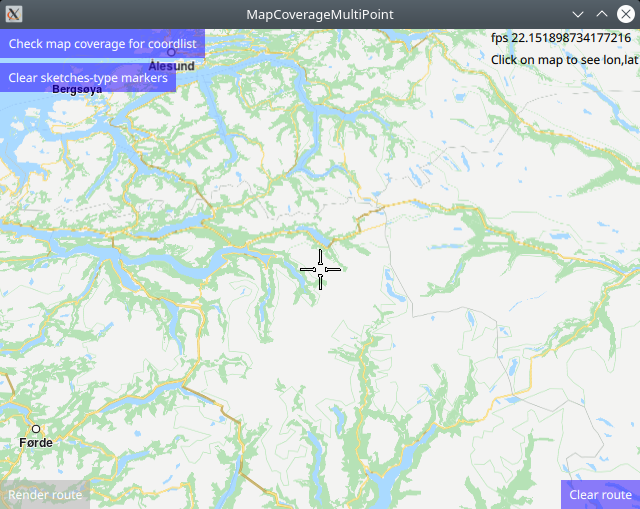
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
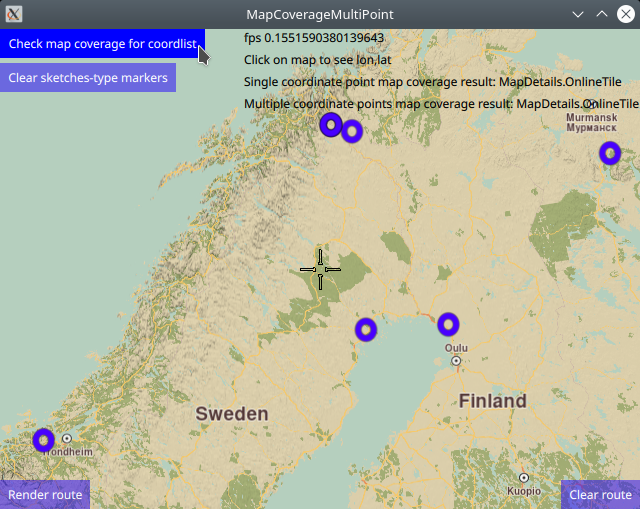
MapCoverageMultiPoint
demonstrates how easy it is to use MapView
to display an interactive map, and add markers programmatically at
specified coordinates, which are displayed as icons on the map.
Then the map coverage is checked for these coordinates, and the
results are displayed on screen.
Also, the markers can be used as waypoints for rendering a route.
There are two types of markers: sketches-type markers and regular markers.
The sketches-type markers cannot be grouped, and all of them are displayed regardless of the zoom level. A custom icon image can be configured for rendering the icons. If no image is configured, a blue filled circle will be used as the icon. These are the types of markers used in this example.
To see an example using both sketches-type (non-grouping) icons,
and grouping marker icons, see the MarkersProgrammatic
example.
Setting the custom icon image for sketches-type markers - see qml.qrc
and main.qml
:
markerRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/violetcircle.png");
Alternatively, instead of using a custom icon image, sketches-type markers can
also be rendered on the map using the predefined red- green- or blue- filled circles,
specified by enum value - see main.qml
:
markerRenderSettings.icon = ServicesManager.createIconFromId(Icon.GreenBall);
The custom icon images, such as png images, optionally with transparency, and map styles must be located in the project directory and configured in the qml.qrc file, shown below:
1<RCC>
2 <qresource prefix="/">
3 <file>main.qml</file>
4 <file>redcircle.png</file>
5 <file>greensquare.png</file>
6 <file>bluetriangle.png</file>
7 <file>violetcircle.png</file>
8 <file>Basic_1_Night_Blues_with_Elevation-1_5_688.style</file>
9 <file>Basic_1_Oldtime_with_Elevation-1_12_657.style</file>
10 </qresource>
11</RCC>
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the MapCoverageMultiPoint example folder and open MapCoverageMultiPoint.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
Single point map coverage¶
1//////////////////////////////////////////////////////////////////////////////////
2//check map coverage for one point - (latitude,longitude) coordinate
3//////////////////////////////////////////////////////////////////////////////////
4let onecoord = ServicesManager.createCoordinates(69.05635,20.55399,0);//lat,lon,alt
5let mapcover = ServicesManager.mapDetails.coverage(onecoord);
6let coverageResult = "Single coordinate point map coverage result: ";
7switch ( mapcover )
8{
9case MapDetails.Unknown:
10 coverageResult = coverageResult + "MapDetails.Unknown"; break;
11case MapDetails.Offline:
12 coverageResult = coverageResult + "MapDetails.Offline"; break;
13case MapDetails.OnlineNoData:
14 coverageResult = coverageResult + "MapDetails.OnlineNoData"; break;
15case MapDetails.OnlineTile:
16 coverageResult = coverageResult + "MapDetails.OnlineTile"; break;
17default:
18 coverageResult = coverageResult + "default case - undefined"; break;
19}
20singleCoordMapCoverage.text = coverageResult;
21console.log(coverageResult);
To check map coverage at one specific lon,lat coordinate,
define the coordinate, onecoord
in this case, and then call
ServicesManager.mapDetails.coverage(onecoord);
, and finally
process the result as shown above.
The coverage result is shown on screen, and also logged in the
console output.
Multiple point map coverage¶
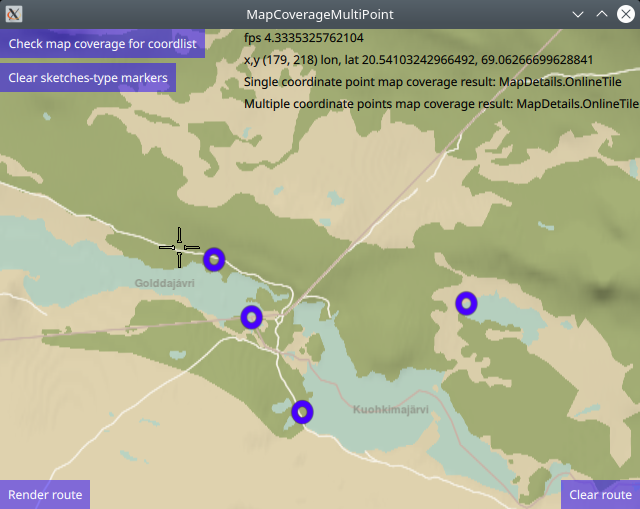
1//////////////////////////////////////////////////////////////////////////////////
2//define a list containing multiple coordinates
3//////////////////////////////////////////////////////////////////////////////////
4let markercoordlist = ServicesManager.createMarker();
5markercoordlist.append(createSketchesPoi(69.05997,20.54865));//lat,lon - no/se/fi border tripoint
6markercoordlist.append(createSketchesPoi(69.06218,20.54471));//lat,lon - no
7markercoordlist.append(createSketchesPoi(69.05635,20.55399));//lat,lon - se
8markercoordlist.append(createSketchesPoi(69.06043,20.57157));//lat,lon - fi
9markercoordlist.append(createSketchesPoi(68.9450, 21.5261 ));//lat,lon - fi
10markercoordlist.append(createSketchesPoi(65.6339, 25.2500 ));//lat,lon - fi
11markercoordlist.append(createSketchesPoi(65.6256, 21.9006 ));//lat,lo - se
12markercoordlist.append(createSketchesPoi(63.3258, 9.4853 ));//lat,lon - no
13markercoordlist.append(createSketchesPoi(68.0924, 33.3895 ));//lat,lon - ru
14//////////////////////////////////////////////////////////////////////////////////
15//check map coverage for multiple coordinates
16//////////////////////////////////////////////////////////////////////////////////
17let mapcovermulti = ServicesManager.mapDetails.coverageCoordList(markercoordlist)
18coverageResult = "Multiple coordinate points map coverage result: ";
19switch ( mapcovermulti )
20{
21case MapDetails.Unknown:
22 coverageResult = coverageResult + "MapDetails.Unknown"; break;
23case MapDetails.Offline:
24 coverageResult = coverageResult + "MapDetails.Offline"; break;
25case MapDetails.OnlineNoData:
26 coverageResult = coverageResult + "MapDetails.OnlineNoData"; break;
27case MapDetails.OnlineTile:
28 coverageResult = coverageResult + "MapDetails.OnlineTile"; break;
29default:
30 coverageResult = coverageResult + "default case - undefined"; break;
31}
32multiCoordsMapCoverage.text = coverageResult;
33console.log(coverageResult);
To check map coverage for a list of lon,lat coordinates,
define the coordinate list using a marker as shown above,
markercoordlist
in this case, and then call
ServicesManager.mapDetails.coverageCoordList(markercoordlist)
,
and finally process the result as shown above.
The coverage result is shown on screen, and also logged in the
console output.
Country code and map coverage¶

The following for loop code block demonstrates how to get the country code
from a lon,lat coordinate using ServicesManager.mapDetails.countryCode()
and how to get country map coverage status from a country code using
ServicesManager.mapDetails.countryCoverage()
as well as the country name
from the country code, and print the results along with the index and lon,lat
coordinates to the application console output window.
1//////////////////////////////////////////////////////////////////////////////////
2// for each coordinate in the list, print in the console output:
3// index, map coverage status, country code, coordinate(lon,lat), country name
4//////////////////////////////////////////////////////////////////////////////////
5let countrycode = "";
6for (let idx = 0; idx < markercoordlist.length; idx++) {
7 countrycode = ServicesManager.mapDetails.countryCode(markercoordlist.get(idx))
8 let mapcountrycover = ServicesManager.mapDetails.countryCoverage(countrycode)
9 coverageResult = "Country coverage result by coordinate point: ";
10 switch ( mapcountrycover )
11 {
12 case MapDetails.Unknown:
13 coverageResult = coverageResult + "MapDetails.Unknown"; break;
14 case MapDetails.Offline:
15 coverageResult = coverageResult + "MapDetails.Offline"; break;
16 case MapDetails.OnlineNoData:
17 coverageResult = coverageResult + "MapDetails.OnlineNoData"; break;
18 case MapDetails.OnlineTile:
19 coverageResult = coverageResult + "MapDetails.OnlineTile"; break;
20 default:
21 coverageResult = coverageResult + "default case - undefined"; break;
22 }
23 console.log("[" + idx + "] " + coverageResult + " " + countrycode + " ("
24 + markercoordlist.get(idx).longitude + ", " + markercoordlist.get(idx).latitude
25 + ") " + ServicesManager.mapDetails.countryName(countrycode))
26}
Rendering a route¶
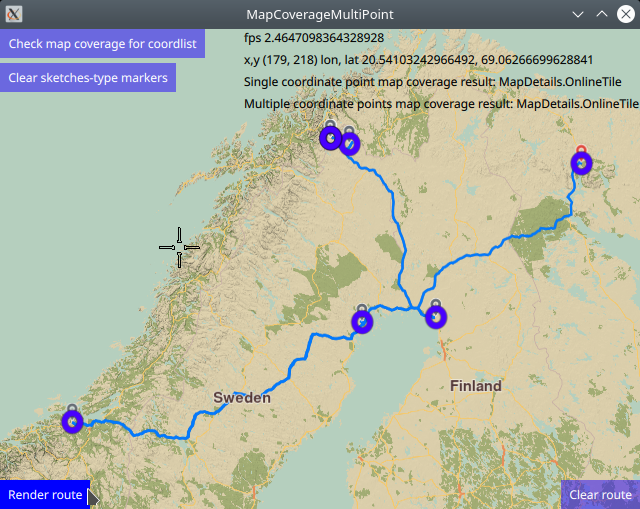
Optionally, a route can be rendered using the markers as waypoints, in the order in which they were added.
1RoutingService {
2 id: routingService
3 type: Route.Type.Fastest
4 transportMode: Route.TransportMode.Car
5 // The list of waypoints is made available to the routing service.
6 waypoints: routingWaypoints
7 onFinished: {
8 map.routeCollection.set(routeList);
9 map.centerOnRouteList(routeList);
10 }
11}
A RoutingService is defined, used in this example only to
compute and render a route plotted using the list of waypoints/landmarks
in the order in which they were added to the list;
this block is not required to autocenter on the landmark group.
Set internet connection to true in the Component.onCompleted
block
for route computation and rendering to work.
Click the Render route
button to compute and render the route.
Click the Clear route
button to remove the route from the map.
Removing sketches-type markers¶
The route and/or sketches-type markers can be removed from the map separately.
1Button {
2 text: "Clear sketches-type markers"
3 onClicked: {
4 routingWaypoints.clear();
5 let sketchlist = map.markerCollection.getExtendedList(MarkerList.Type.Point);
6 sketchlist.clear();
7 }
8}
Removing the sketches-type markers is done by again getting a pointer
to the built-in sketches-type marker list, and using the clear()
function.
The corresponding routingWaypoints
list, used to render the
route or center on the group of markers, or both, is also cleared.
The same set of coordinates is in each of these lists,
so both must be cleared.