Map At Position¶
In this guide you will learn how to find out which map (which may be of a region or country) contains a given (longitude, latitude) coordinate, also the size in bytes of that map, and whether it is already downloaded or not.
Find map containing specified coordinate¶
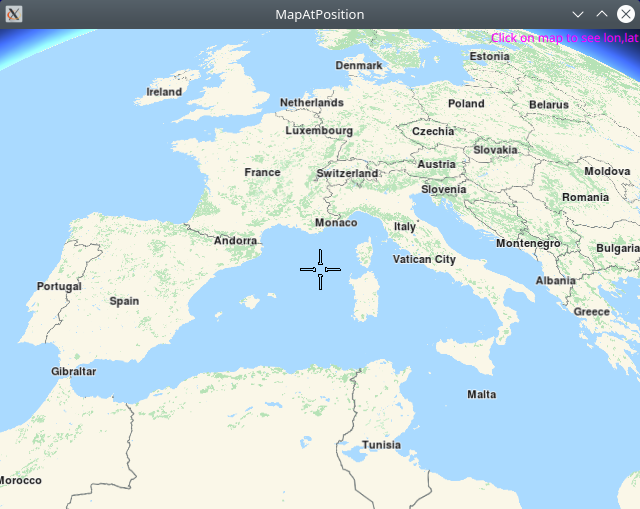
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
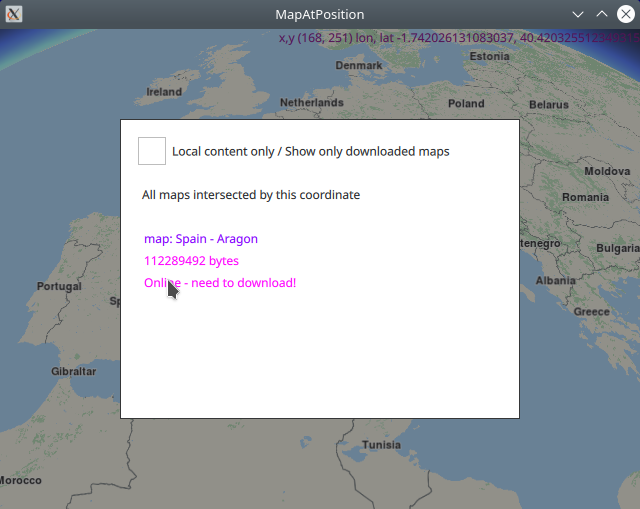
MapAtPosition
demonstrates how easy it is to use MapView
to display an interactive map, and query the ContentStore
with a
given (longitude, latitude) coordinate pair, to find out which map
contains that location.
The resulting list of maps, usually 1, along with their sizes in bytes, containing the given coordinate, are displayed in a popup.
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the MapAtPosition example folder and open MapAtPosition.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
Map containing specified position¶
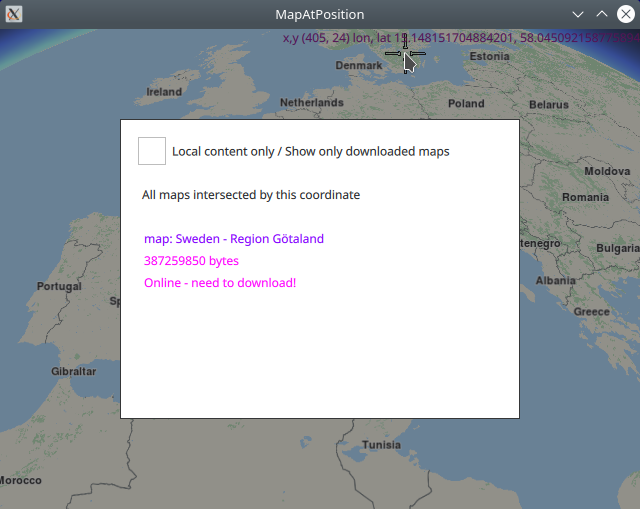
1/////////////////////////////////////////////////////////////////////////////////
2//display x,y pixel position and lon,lat coordinate of clicked position on map
3//////////////////////////////////////////////////////////////////////////////////
4TapHandler {
5 grabPermissions: PointerHandler.CanTakeOverFromAnything
6 onTapped: {
7 let coordinates = map.wgsForScreen(point.pressPosition);
8 console.log("x,y (" + point.pressPosition.x + ", " + point.pressPosition.y + ")"
9 + " lon, lat " + coordinates.longitude + ", " + coordinates.latitude);
10 lonlatPositionDisplay.text = "x,y (" + point.pressPosition.x + ", " + point.pressPosition.y + ")"
11 + " lon, lat " + coordinates.longitude + ", " + coordinates.latitude;
12 lonlatPositionDisplay.color = "#800080"
13 contentStore.intersectsCoordinate(coordinates.latitude, coordinates.longitude);
14 flag.gotCoord = true;
15 }
16}
The TapHandler
block of code within the MapView
block detects where the
user clicked on the map, and converts the x,y screen coordinates to longitude,latitude
coordinates on the globe using the wgsForScreen()
function, and then queries the
online content store using the intersectsCoordinate()
function, to find out which
map(s) contain the specified coordinate.
This is done using the intersectsCoordinate() function from the ContentStore
The result is received asynchronously via the onStatusChanged
signal below.
A ContentStore
instance which listens for the onStatusChanged
signal
is defined, along with a connection to the online content store,
(which has maps, voices and styles), and the update()
function is called
in the Component.onCompleted:
block.
1Component.onCompleted: {
2 //! [Set token safely]
3 ServicesManager.settings.token = __my_secret_token;
4 //! [Set token safely]
5
6 ServicesManager.logLevel = ServicesManager.Error;
7 ServicesManager.settings.allowInternetConnection = true; // enable connection to online services
8
9 updater.autoApplyWhenReady = true;
10 updater.update();
11}
12Connections {
13 target: updater
14 onFinished: {
15 if (result === GeneralMagic.Result.Ok || result === GeneralMagic.Result.UpToDate)
16 contentStore.update();
17 else
18 console.error("Content update failed");
19 }
20}
21ContentStore {
22 id: contentStore
23 type: ContentItem.Type.RoadMap //content.type
24 onStatusChanged: {
25 if ( flag.gotCoord )
26 intersectedMapsPopup.open()
27 }
28}
29Item {
30 id: flag
31 property bool gotCoord: false;
32}
A flag is also defined to indicate when a coordinate was selected interactively on the map by a user click, because the status change signal may occur for other reasons as well. The flag is initialized to false, set to true when the user clicks on the map to select a coordinate, and set to false again when the user clicks outside the popup showing the resulting list of map(s) containing the given coordinate, and thus the popup is closed.
When onStatusChanged
signal is received, the content store internal map list
is populated with the results, which in this case is the list of maps (usually 1)
containing the specified coordinate. Note that this is a list of maps,
not necessarily of countries.
A map may contain an entire country, or only a part, or region, of a country.
Displaying the list of maps¶
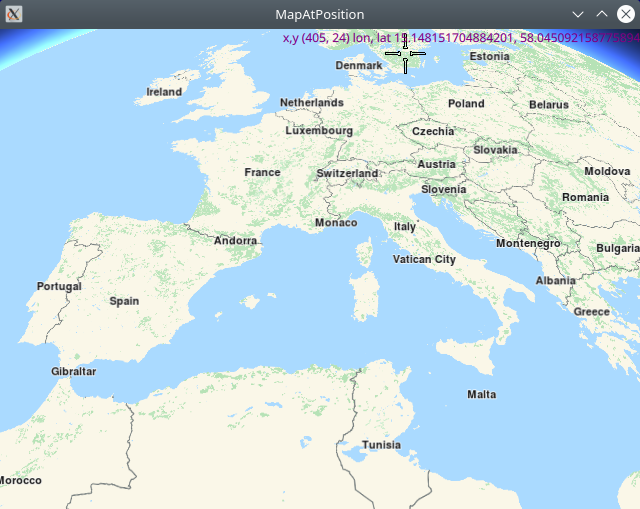
The list of maps traversed by the given route is displayed in a popup and is scrollable. The size in bytes of each map is also shown, along with whether the map is already downloaded or not.
The popup uses the contentStore
model to display the data populated in the
asynchronous reply. When the onStatusChanged
signal is received, the popup
is invoked to show the results using the intersectedMapsPopup.open()
function.
1Popup {
2 //////////////////////////////////////////////////////////////////////////////////
3 //list of maps covering this coordinate shown in interactive popup - click outside to close;
4 //////////////////////////////////////////////////////////////////////////////////
5 id: intersectedMapsPopup
6 modal: true
7 focus: true
8 closePolicy: Popup.CloseOnEscape | Popup.CloseOnPressOutside
9 width: 400
10 height: 300
11 anchors.centerIn: parent
12 ColumnLayout {
13 anchors.fill: parent
14 anchors.top: parent.TopLeft
15 RowLayout {
16 Layout.fillWidth: true
17 CheckBox {
18 id: localMapsCheckBox
19 text: "Local content only / Show only downloaded maps"
20 onCheckedChanged: storeList.model.localContentOnly = checked
21 }
22 }
23 RowLayout {
24 spacing: 10
25 Layout.margins: 10
26 Label {
27 text: localMapsCheckBox.checked ? "Maps intersected by this coordinate, which are also downloaded"
28 : "All maps intersected by this coordinate"
29 }
30 Button {
31 visible: contentStore.status !== GeneralMagic.Result.Ok
32 text: "Update failed, refresh?"
33 onClicked: contentStore.update()
34 }
35 }
36 Item {
37 Layout.fillHeight: true
38 Layout.fillWidth: true
39 ColumnLayout {
40 anchors.fill: parent
41 ListView {
42 id: storeList
43 clip: true
44 Layout.fillHeight: true
45 Layout.fillWidth: true
46 model: contentStore
47 delegate:
48 ItemDelegate {
49 id: control
50 width: storeList.width
51 contentItem: RowLayout {
52 id: resultmaprow
53 ColumnLayout {
54 Layout.fillHeight: true
55 Layout.fillWidth: true
56 Text {
57 font.pixelSize: 12
58 color: "#8000ff"
59 text: "map: " + modelData.name
60 }
61 Text {
62 font.pixelSize: 12
63 color: modelData.completed ? "#008080" : "#ff00ff"
64 text: modelData.totalSize + " bytes"
65 }
66 Text {
67 font.pixelSize: 12
68 color: modelData.completed ? "#008080" : "#ff00ff"
69 text: modelData.completed ? "Local / downloaded / ready"
70 : "Online - need to download!"
71 }
72 }
73 TapHandler {
74 target: resultmaprow
75 onTapped: {
76 intersectedMapsPopup.close()
77 flag.gotCoord = false;
78 }
79 }
80 }
81 }
82 }
83 }
84 }
85 }
86}
See the ContentDownload
example to see how to download maps from the content store.