Map Coordinates¶
In this guide you will learn how to use MapView
to display an interactive map, and get and display the
longitude and latitude coordinates of any point
tapped/clicked on the map by the user.
Coordinates at user click position¶
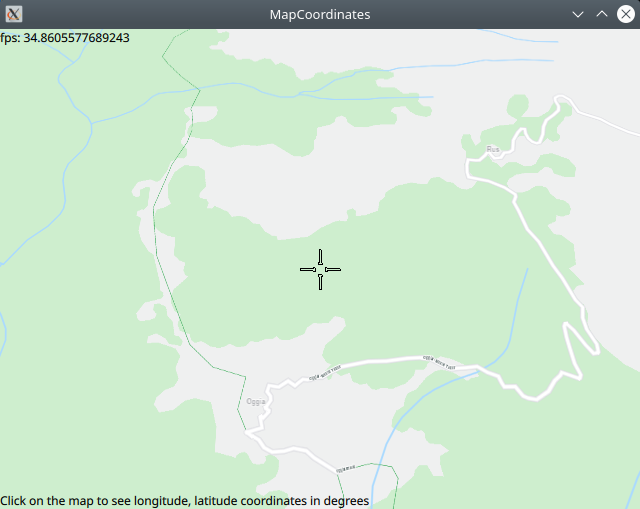
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
MapCoordinates
demonstrates how easy it is to get the
longitude and latitude coordinates of a user-selected point
on an interactive map displayed by MapView
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the MapCoordinates example folder and open MapCoordinates.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
1import QtQuick 2.12
2import QtQuick.Window 2.12
3import GeneralMagic 2.0
4import FpsCounter 1.0
In main.qml, we need to import the GeneralMagic QML plugin.
FpsCounter is used to display the rendering speed in frames per second.
Next, we need to make sure we allow online access,
and that we are using the latest data by setting allow internet
connection to true:
ServicesManager.settings.allowInternetConnection = true;
as seen in the code below.
1Component.onCompleted: {
2 //! [Set token safely]
3 ServicesManager.settings.token = __my_secret_token;
4 //! [Set token safely]
5
6 ServicesManager.logLevel = ServicesManager.Error;
7 ServicesManager.settings.allowInternetConnection = true;
8
9 updater.autoApplyWhenReady = true;
10 updater.update();
11}
In the Component.onCompleted:
block, the
b __my_secret_token property in the above QML code is set in C++
as shown below. In this example, in main.cpp
, replace the
"YOUR_TOKEN"
string with your actual General Magic Maps API Key.
1// C++ code
2//! [Set API Key token safely]
3// go to https://developer.magiclane.com to get your token
4engine.rootContext()->setContextProperty("__my_secret_token", "YOUR_TOKEN");
5//! [Set API Key token safely]
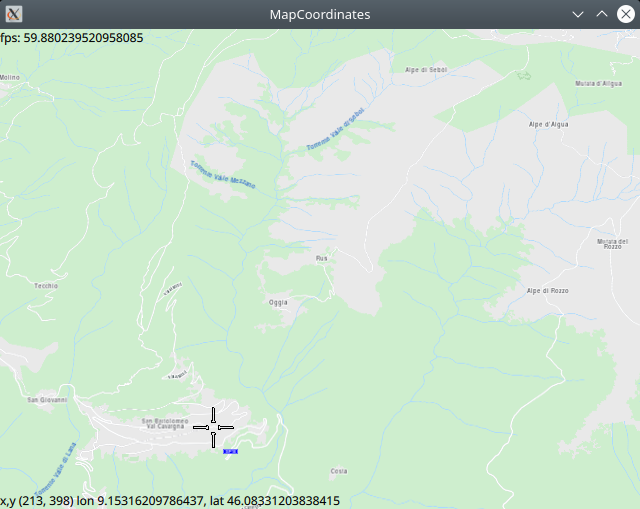
1FpsCounter {
2 id: fpsCounter
3}
The FpsCounter
is updated from C++, and is defined in
fpscounter.h
included in this example.
It has a readable qml property, fps
,
1Q_PROPERTY(double fps READ fps NOTIFY fpsChanged)
which is displayed in the text field in the upper left corner of the viewport, as seen in the code below.
1MapView {
2 id: map
3 anchors.fill: parent
4 viewAngle: 25
5 zoomLevel: 69
6 viewPerspective: MapView.ViewPerspective.View3D
7 buildingsVisibility: MapView.BuildingsVisibility.Show3D
8 detailsQualityLevel: MapView.DetailsQualityLevel.Medium
9 gestures: MapView.Gesture.Pan
10 | MapView.Gesture.PanEnableVelocity
11 | MapView.Gesture.Pinch
12 | MapView.Gesture.Rotate
13 | MapView.Gesture.Tilt
14 Text {
15 anchors.left: parent.left
16 anchors.top: parent.top
17 text: "fps: "+fpsCounter.fps
18 }
19 Text {
20 id: lonlat
21 anchors.left: parent.left
22 anchors.bottom: parent.bottom
23 text: "Click on the map to see longitude, latitude coordinates in degrees"
24 }
25 TapHandler {
26 grabPermissions: PointerHandler.CanTakeOverFromAnything
27 onTapped: {
28 let coordinates = map.wgsForScreen(point.pressPosition);
29 console.log("x,y ("+point.pressPosition.x+", "+point.pressPosition.y+")"
30 +" lon "+coordinates.longitude+", lat "+coordinates.latitude);
31 lonlat.text = "x,y ("+point.pressPosition.x+", "+point.pressPosition.y+")"
32 +" lon "+coordinates.longitude+", lat "+coordinates.latitude;
33 }
34 }
35}
In the MapView
block, the map settings, such as rendering 3D buildings,
and user input gestures to be processed, such as pan, pinch, or rotate,
are specified. MapView.Gesture.PanEnableVelocity
enables swipe.
Next, the top left text field displays the fps property of the fpsCounter,
where fpsCounter
is the given id of the {FpsCounter}.
The bottom left text field displays the viewport-relative x,y position,
in pixels, where the viewport top left corner is (0,0), and the
corresponding longitude and latitude coordinates, in degrees, of the
point on the map clicked by the user. To do this, a TapHandler
is
defined to handle the tap/click gesture in the onTapped
block.
The conversion from 2D viewport x,y coordinates to longitude,latitude
coordinates is done by the map.wgsForScreen
function, where
map
is the id of the MapView
block above: id: map
let coordinates = map.wgsForScreen(point.pressPosition);
The onTapped
block updates the text field in the viewport
by setting its text property, using the id of the
text field: id: lonlat
, thus: lonlat.text = "";
This information is also printed in the Qt application output window
using console.log("");
and the output appears like this:
qml: x,y (213, 398) lon 9.15316209786437, lat 46.08331203838415