Play and record navigation GPS log¶
Play back a previously recorded or generated GPS log as input and record a GPS log as output.
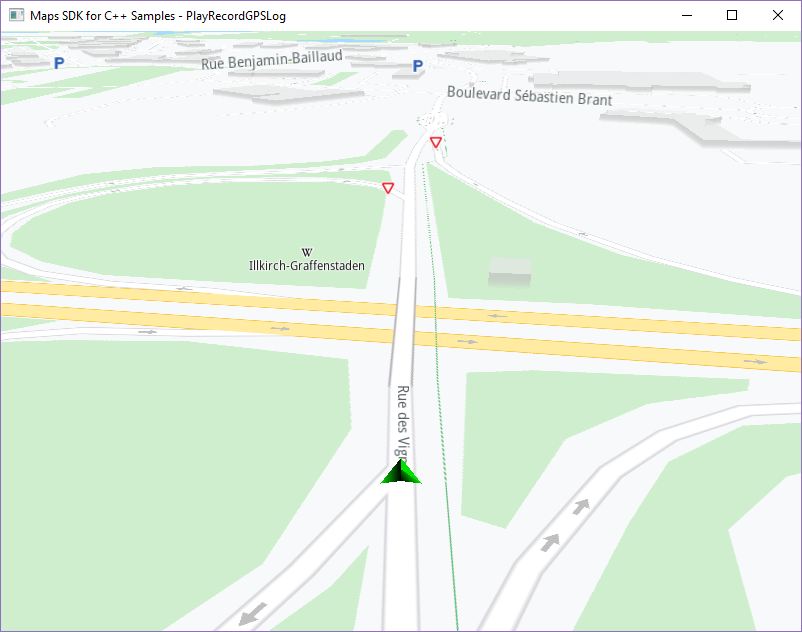
Use case¶
Demonstrate playback of a previously recorded or generated GPS log, as well as recording a GPS log.
How to use the sample¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for C++ archive file for Linux or WindowsNavigation is simulated along the route previously recorded or generated in the input GPS log; an output GPS log with the .gm extension is written.
How it works¶
Create an instance of
Environment
and set your API key token:
1Environment& env = Environment::GetInstance();
2
3//
4// Project API token available at:
5// https://developer.magiclane.com/api/projects
6//
7std::string projectApiToken = ""; //YOUR_TOKEN
The SDK is initialized with your API key token string and the log file path, where to write the application logs. Note that
logFilePath
is not initialized by default, which means that no logs are written. The logFilePath is initialized with the first command line argument, if any.
1std::string logFilePath;
2if ( argc > 1 )
3 logFilePath = std::string(argv[1]);
4
5env.InitSDK( projectApiToken, logFilePath.c_str() );
The project path is obtained, to get an absolute path to the
strasbourg.nmea
input GPS log file. The resources path, as defined inEnvironment.cpp
, is the path containing theData
andExamples
subdirectories.
1std::string sdkResrcPath = Environment::GetResourcesPath();
2std::string projectRelativePath = "\\Examples\\Maps-SDK-Examples-for-Cpp\\Examples\\RoutesAndNavigation\\PlayRecordGPSLog\\";
3std::string projectAbsolutePath = sdkResrcPath + projectRelativePath;
4std::string inputGPSlogFile = projectAbsolutePath + "strasbourg.nmea";
Create a
MapViewListener
,OpenGLContext
with the name of the app, the name of this example in this case, and aMapView
. The path for writing the output GPS log files is also specified, thegpslogs
directory in this case, which is set in the same directory as theData
andExamples
directories, so it should be created there before running the example.
1MapViewListenerImpl listener;
2auto oglContext = env.ProduceOpenGLContext("PlayRecordGPSLog");
3gem::StrongPointer<gem::MapView> mapView = gem::MapView::produce(oglContext, &listener);
4gem::String logsdir(sdkResrcPath + "gpslogs");
Create a
RecorderConfiguration
withPosition
andImprovedPosition
selected for recording in aDataTypeList
:
1gem::sense::DataTypeList datatypes;
2datatypes.push_back(gem::sense::EDataType::Position);
3datatypes.push_back(gem::sense::EDataType::ImprovedPosition);
4gem::RecorderConfiguration recsrcconfig(logsdir, datatypes);
Set the chunk duration to be recorded to 60 sec in
RecorderConfiguration
, as 30 sec is the minimum. Create aDataSourcePtr
using the input GPS log filename, and then create aRecorder
using the recorder configuration and data source.
1recsrcconfig.chunkDurationSeconds = 60;
2recsrcconfig.bContinuousRecording = true;
3recsrcconfig.deleteOlderThanKeepMin = false;
4recsrcconfig.keepMinSeconds = 3600;
5std::pair<gem::sense::DataSourcePtr, int> dataSource = gem::sense::produceLogDataSource(inputGPSlogFile.c_str());
6auto rec = gem::Recorder::produce(recsrcconfig, dataSource.first);
Set the data source in the
PositionService
singleton for GPS log playback. This will move the green arrow on the map along the path it followed when the GPS log was recorded or generated.Start recording the playback simulation, and start following the simulated position. This will fly the camera to the green arrow and start tracking/following the green arrow as it moves on the map.
1if (dataSource.first)
2{
3 gem::PositionService().setDataSource(dataSource.first);
4 rec.get()->startRecording();
5}
6
7WAIT_TIME_OUT(1000);
8
9mapView->startFollowingPosition();
Stop recording when the window is closed to save the output GPS log file.
1WAIT_UNTIL_WINDOW_CLOSE();
2
3rec.get()->stopRecording();