Search Along Route ¶
Setup ¶
Download the
SearchAlongRoute
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|
How it works ¶
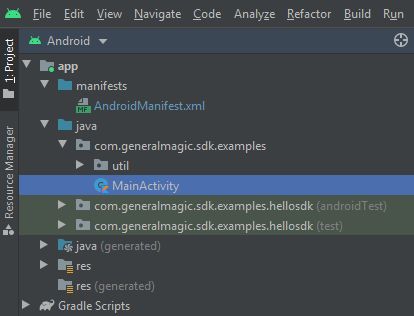
You can open the MainActivity.kt file to see how simulated navigation along a computed route with user-triggered search along route works.
1private val navigationService = NavigationService()
A
NavigationService()
is instantiated, which carries out
both simulated navigation and real navigation.
1private val searchService = SearchService(
2 onStarted = {
3 progressBar.visibility = View.VISIBLE
4 },
5 onCompleted = onCompleted@{ results, errorCode, _ ->
6 progressBar.visibility = View.GONE
7 when (errorCode) {
8 GemError.NoError -> {
9 // Display results in AlertDialog
10 onSearchCompleted(results)
11 }
12 GemError.Cancel -> {
13 // The search action was canceled.
14 }
15 else -> {
16 // There was a problem in the search operation.
17 Toast.makeText(
18 this@MainActivity,
19 "Search service error: ${GemError.getMessage(errorCode)}",
20 Toast.LENGTH_SHORT
21 ).show()
22 }
23 }
24 }
25)
A
SearchService()
is instantiated, which carries out
on-demand search along the route.
1private val navigationListener: NavigationListener = NavigationListener.create(
2 onNavigationStarted = {
3 SdkCall.execute {
4 gemSurfaceView.mapView?.let { mapView ->
5 mapView.preferences?.enableCursor = false
6 getNavRoute()?.let { route ->
7 mapView.presentRoute(route)
8 // Make the search button visible and
9 // add a click listener to do the search.
10 Util.postOnMain {
11 searchButton.visibility = View.VISIBLE
12 searchButton.setOnClickListener { searchAlongRoute(route) }
13 }
14 }
15 enableGPSButton()
16 mapView.followPosition()
17 }
18 }
19 }
20)
NavigationListener
receives event updates (notifications) from
the navigation service, during navigation or simulation on a route, such as
when navigation starts or when the destination is reached,
or when the route to the desired destination
has been recomputed, because a detour away from the original route was taken.
onNavigationStarted
listener is instantiated in this case.
1private val routingProgressListener = ProgressListener.create(
2 onStarted = {
3 progressBar.visibility = View.VISIBLE
4 },
5 onCompleted = { _, _ ->
6 progressBar.visibility = View.GONE
7 },
8 postOnMain = true
9)
mapView.followPosition()
causes
the camera to follow the green arrow.
|
|||
1private fun enableGPSButton() {
2 // Set actions for entering/ exiting following position mode.
3 gemSurfaceView.mapView?.apply {
4 onExitFollowingPosition = {
5 followCursorButton.visibility = View.VISIBLE
6 }
7 onEnterFollowingPosition = {
8 followCursorButton.visibility = View.GONE
9 }
10 // Set on click action for the GPS button.
11 followCursorButton.setOnClickListener {
12 SdkCall.execute { followPosition() }
13 }
14 }
15}
enableGPSButton()
causes a round purple button to appear in the lower
right corner of the screen, whenever the simulation is active and
the camera is not following the green arrow. If the user pushes this button,
the
followPosition()
function is called, and thus the camera
starts to follow the green arrow once again.
1private fun startSimulation() = SdkCall.execute {
2 val waypoints = arrayListOf(
3 Landmark("London", 51.5073204, -0.1276475),
4 Landmark("Paris", 48.8566932, 2.3514616)
5 )
6 navigationService.startSimulation(waypoints, navigationListener, routingProgressListener)
7}
1private fun searchAlongRoute(route: Route) = SdkCall.execute {
2 // Set the maximum number of results to 25.
3 searchService.preferences.maxMatches = 25
4 // Search Gas Stations along the route.
5 searchService.searchAlongRoute(route, EGenericCategoriesIDs.GasStation)
6}
onNavigationStarted
listener causes the search along route button
to appear in the lower left corner of the viewport while navigation is active.
searchAlongRoute()
function
is called and set to return up to 25 gas stations along the route.
1private fun onSearchCompleted(results: ArrayList<Landmark>) {
2 val builder = AlertDialog.Builder(this)
3 val convertView = layoutInflater.inflate(R.layout.dialog_list, null)
4 convertView.findViewById<RecyclerView>(R.id.list_view).apply {
5 layoutManager = LinearLayoutManager(this@MainActivity)
6 addItemDecoration(DividerItemDecoration(
7 applicationContext,
8 (layoutManager as LinearLayoutManager).orientation
9 ))
10 setBackgroundResource(R.color.white)
11 val lateralPadding = resources.getDimension(R.dimen.bigPadding).toInt()
12 setPadding(lateralPadding, 0, lateralPadding, 0)
13 adapter = CustomAdapter(results)
14 }
15 builder.setView(convertView)
16 builder.create().show()
17}
onCompleted
listener in the
searchService
calls the
onSearchCompleted()
function when the user-triggered search is finished.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 progressBar = findViewById(R.id.progressBar)
5 gemSurfaceView = findViewById(R.id.gem_surface)
6 searchButton = findViewById(R.id.search_button)
7 followCursorButton = findViewById(R.id.followCursor)
8 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
9 if (!isReady) return@onMapDataReady
10 // Defines an action that should be done when
11 // the world map is ready (updated / loaded).
12 startSimulation()
13 }
14 SdkSettings.onApiTokenRejected = {
15 Toast.makeText(this@MainActivity, "TOKEN REJECTED",
16 Toast.LENGTH_SHORT).show()
17 }
18 if (!Util.isInternetConnected(this)) {
19 Toast.makeText(this, "You must be connected to internet!",
20 Toast.LENGTH_LONG).show()
21 }
22}
MainActivity
overrides the
onCreate()
function which checks that
internet access is available, and then, when the map is instantiated
and ready, starts the simulation:
startSimulation()
.
searchButton
.