GPX Route Simulation ¶
In this guide you will learn how to load a GPX route, render it on the map, and then simulate navigation along that route.
Setup ¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive file
Download the
GPXRouteSimulation
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|
|
A GPX route is loaded and rendered on the map. Navigation along the GPX route is simulated. If a pan occurs, a button appears in the lower right corner of the screen. Pressing this button resumes following/tracking the green navigation simulation arrow along the route.
How it works ¶
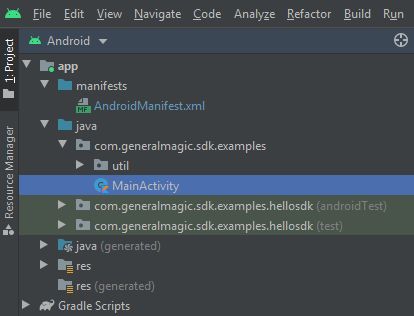
You can open the MainActivity.kt file to see how the GPX route is imported and rendered on the map.
class
MainActivity
:
AppCompatActivity()
,
an instance of
val
routingService
=
RoutingService()
is created.
override
fun
onCreate()
calls the
calculateRouteFromGPX()
function after the map is loaded.
1private fun calculateRouteFromGPX() = SdkCall.execute {
2 val gpxAssetsFilename = "gpx/test_route.gpx"
3
4 // Opens GPX input stream.
5 val input = applicationContext.resources.assets.open(gpxAssetsFilename)
6
7 // Produce a Path based on the data in the buffer.
8 val track = Path.produceWithGpx(input/*.readBytes()*/) ?: return@execute
9
10 // Set the transport mode to bike and calculate the route.
11 routingService.calculateRoute(track, ERouteTransportMode.Bicycle)
12}
"gpx/test_route.gpx"
which is found in the
assets/
directory of this example.
track
=
Path.produceWithGpx(input)
routingService.calculateRoute(track,
ERouteTransportMode.Bicycle)
Inside the
val
routingService
=
RoutingService()
, the simulation
is started automatically when the route computation is completed, using
the first (index 0) route
routes[0]
in the resulting route list:
1GemError.NoError -> {
2 val route = routes[0]
3 SdkCall.execute {
4 navigationService.startSimulationWithRoute(
5 route,
6 navigationListener,
7 routingProgressListener
8 )
9 }
10}
val
navigationListener:
NavigationListener
is to detect when a user-driven pan of the map occurred.
1private val navigationListener: NavigationListener
2= NavigationListener.create(
3 onNavigationStarted = {
4 SdkCall.execute {
5 gemSurfaceView.mapView?.let { mapView ->
6 mapView.preferences?.enableCursor = false
7 navRoute?.let { route ->
8 mapView.presentRoute(route)
9 }
10 enableGPSButton()
11 mapView.followPosition()
12 }
13 }
14 }
15)