Hello SDK ¶
In this guide you will learn how to create a Hello World app using the Maps SDK for Android.
Setup ¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive file
Download the
HelloSDK
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|||
How it works ¶
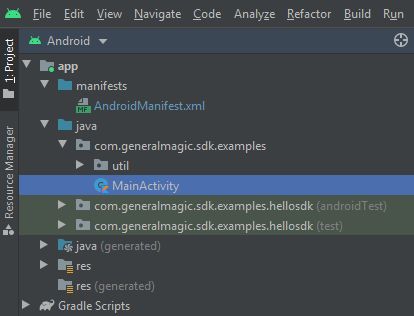
You can open the MainActivity.kt file to see how to create an empty app and import the Maps SDK for Android.
1package com.magiclane.sdk.examples.hellosdk
2
3import android.os.Bundle
4import android.widget.Toast
5import androidx.appcompat.app.AppCompatActivity
6import com.magiclane.sdk.core.GemSdk
7import com.magiclane.sdk.core.SdkSettings
8import kotlin.system.exitProcess
9
10class MainActivity : AppCompatActivity() {
11 override fun onCreate(savedInstanceState: Bundle?) {
12 super.onCreate(savedInstanceState)
13 setContentView(R.layout.activity_main)
14 SdkSettings.onApiTokenRejected = {
15 Toast.makeText(this, "TOKEN REJECTED", Toast.LENGTH_LONG).show()
16 }
17 if (!GemSdk.initSdkWithDefaults(this)) {
18 // The SDK initialization was not completed.
19 finish()
20 }
21 }
22 override fun onDestroy() {
23 super.onDestroy()
24 // Release the SDK.
25 GemSdk.release()
26 }
27 override fun onBackPressed() {
28 finish()
29 exitProcess(0)
30 }
31}
import
com.magiclane.sdk.core.GemSdk
import
com.magiclane.sdk.core.SdkSettings
MainActivity
overrides the
onCreate
function which
GemSdk.initSdkWithDefaults(this)
setContentView(R.layout.activity_main)
then displays a “Hello World!” message on a blank viewport,
as defined in
res/layout/activity_main.xml
in the project.