External Position Source Navigation ¶
Setup ¶
Download the
ExternalPositionSourceNavigation
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|||
How it works ¶
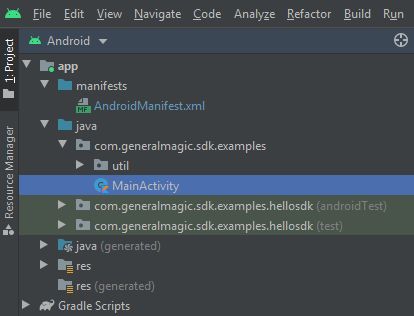
You can open the MainActivity.kt file to see how the external position source navigation works.
1SdkSettings.onMapDataReady = { mapReady ->
2 if (mapReady)
3 {
4 var externalDataSource: ExternalDataSource?
5 var index = 0
6 val positions = arrayOf(Pair(48.133931, 11.582914),
7 Pair(48.134015, 11.583203),
8 Pair(48.134057, 11.583348),
9 Pair(48.134085, 11.583499),
10 ...
MainActivity
overrides the
onCreate
function, in which an array of positions
is defined, to be used as the external position source for navigation.
getBearing
,
getDistanceOnGeoid
,
getSpeed
,
getEta()
,
getRtt()
,
getRtd()
.
1SdkCall.execute {
2 positionService = PositionService()
3 externalDataSource = DataSourceFactory.produceExternal(arrayListOf(EDataType.Position))
4 externalDataSource?.start()
5 positionListener = PositionListener { position: PositionData ->
6 if (position.isValid())
7 {
8 navigationService.startNavigation(
9 Landmark("Poing", 48.17192581, 11.80789822),
10 navigationListener,
11 routingProgressListener
12 )
13 positionService.removeListener(positionListener)
14 }
15 }
16 positionService.dataSource = externalDataSource
17 positionService.addListener(positionListener)
18 externalDataSource?.let { dataSource ->
19 fixedRateTimer("timer", false, 0L, 1000) {
20 SdkCall.execute {
21 val externalPosition
22 = ExternalPosition.produceExternalPosition(System.currentTimeMillis(),
23 positions[index].first,
24 positions[index].second,
25 -1.0,
26 getBearing(),
27 getSpeed())
28 externalPosition?.let { pos ->
29 dataSource.pushData(pos)
30 }
31 index++
32 if (index == positions.size)
33 {
34 index = 0
35 }
36 }
37 }
38 }
39}
externalDataSource
is instantiated for the
EDataType.Position
,
and a
positionListener
is instantiated with the array of position coordinates
previously defined. Both of these instances are set in the
positionService
.
fixedRateTimer
timer is set to read a position from the array every second
(1000 milliseconds), and update the navigation simulation. Although the
positions are read at a steady rate, simulation travel speed can vary, as
subsequent positions may be closer or further from each other.