Map Compass ¶
Setup ¶
Download the
MapCompass
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|
|
|
How it works ¶
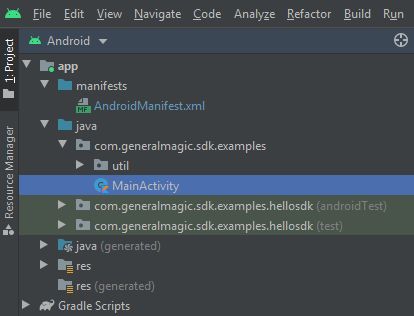
You can open the MainActivity.kt file to see how the map compass works.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4
5 surfaceView = findViewById(R.id.gem_surface)
6 compass = findViewById(R.id.compass)
7 btnEnableLiveHeading = findViewById(R.id.btnEnableLiveHeading)
8
9 // start stop btn
10 buttonAsStart(this, btnEnableLiveHeading)
11
12 btnEnableLiveHeading.setOnClickListener {
13 isLiveHeadingEnabled.set(!isLiveHeadingEnabled.get())
14 if (isLiveHeadingEnabled.get()) {
15 buttonAsStop(this, btnEnableLiveHeading)
16 } else {
17 buttonAsStart(this, btnEnableLiveHeading)
18 }
19 Toast.makeText(
20 this, "Live heading update, enabled=$isLiveHeadingEnabled ",
21 Toast.LENGTH_SHORT
22 ).show()
23 GemCall.execute {
24 if (isLiveHeadingEnabled.get()) {
25 startLiveHeading()
26 } else {
27 stopLiveHeading()
28 }
29 }
30 }
MainActivity
overrides the
onCreate
function which defines the
buttonAsStart
/
buttonAsStop
button used to turn the compass on or off,
as well as color the button green / red, respectively, and a listener for that button,
btnEnableLiveHeading.setOnClickListener
.
startLiveHeading()
or
stopLiveHeading()
to start or stop reading
the live compass sensor data from the device, by adding or removing a listener
to the compass data source.
1private fun startLiveHeading() = GemCall.execute {
2 dataSource = DataSourceFactory.produceLive()
3
4 // start listening for compass data
5 dataSource?.addListener(object : DataSourceListener() {
6 override fun onNewData(dataType: EDataType) {
7 GemCall.execute {
8 dataSource?.getLatestData(dataType)?.let {
9
10 // smooth new compass data
11 val heading = headingSmoother.update(CompassData(it).heading)
12
13 // update map view based on the recent changes
14 surfaceView.mapView?.preferences?.rotationAngle = heading
15 }
16 }
17 }
18 }, EDataType.Compass)
19}
mapView
to rotate the map accordingly, when the device is rotated.
1private fun stopLiveHeading() = GemCall.execute {
2 dataSource?.release()
3 dataSource = null
4}