Overlapped Maps ¶
Setup ¶
Download the Maps & Navigation SDK for Android archive file
Download the
OverlappedMaps
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|
How it works ¶
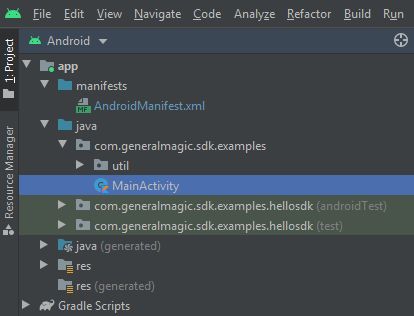
You can open the MainActivity.kt file to see how an interactive map inset is rendered over another interactive map.
1class MainActivity : AppCompatActivity() {
2 private lateinit var gemSurfaceView: GemSurfaceView
3 private var secondMapView: MapView? = null
4
5 override fun onCreate(savedInstanceState: Bundle?) {
6 super.onCreate(savedInstanceState)
7 setContentView(R.layout.activity_main)
8
9 ////////////////////////////////////////////
10 // This code block adds the small inset map:
11 gemSurfaceView = findViewById(R.id.gem_surface)
12 gemSurfaceView.onDefaultMapViewCreated = {
13 gemSurfaceView.gemScreen?.let { screen ->
14 val secondViewRect = RectF(0.0f, 0.0f, 0.5f, 0.5f)
15 secondMapView = MapView.produce(screen, secondViewRect, null, true)
16 }
17 }
18 ////////////////////////////////////////////
19
20 SdkSettings.onApiTokenRejected = {
21 Toast.makeText(this@MainActivity,
22 "TOKEN REJECTED", Toast.LENGTH_SHORT).show()
23 }
24 if (!Util.isInternetConnected(this)) {
25 Toast.makeText(this, "You must be connected to internet!",
26 Toast.LENGTH_LONG).show()
27 }
28 }
29 override fun onDestroy() {
30 super.onDestroy()
31
32 // Deinitialize the SDK.
33 GemSdk.release()
34 }
35 override fun onBackPressed() {
36 finish()
37 exitProcess(0)
38 }
39}
MainActivity
overrides the
onCreate
function, which checks that
internet access is available, and calls the
findViewById()
function to find
the surface on which the default map is rendered.
gemSurfaceView.onDefaultMapViewCreated
=
{
...
}