Marker Collection Display Icon ¶
Setup ¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive file
Download the
MarkerCollectionDisplayIcon
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|||
How it works ¶
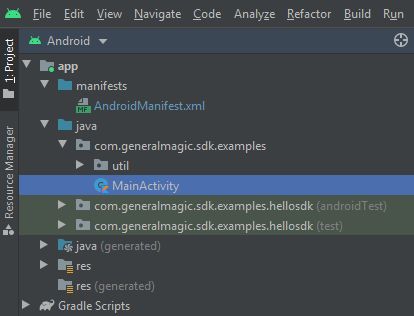
You can open the MainActivity.kt file to see how rendering icons on a map to indicate POIs works.
1private fun getBitmap(drawableRes: Int): Bitmap? {
2 val drawable = if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
3 ResourcesCompat.getDrawable(resources, drawableRes, theme)
4 } else {
5 ContextCompat.getDrawable(this, drawableRes)
6 }
7 drawable ?: return null
8 val canvas = Canvas()
9 val bitmap = Bitmap.createBitmap(
10 drawable.intrinsicWidth,
11 drawable.intrinsicHeight,
12 Bitmap.Config.ARGB_8888
13 )
14 canvas.setBitmap(bitmap)
15 drawable.setBounds(0, 0, drawable.intrinsicWidth, drawable.intrinsicHeight)
16 drawable.draw(canvas)
17 return bitmap
18}
1private fun toPngByteArray(bmp: Bitmap): ByteArray {
2 val stream = ByteArrayOutputStream()
3 bmp.compress(Bitmap.CompressFormat.PNG, 100, stream)
4 val byteArray: ByteArray = stream.toByteArray()
5 bmp.recycle()
6 return byteArray
7}
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 binding = ActivityMainBinding.inflate(layoutInflater)
4 setContentView(binding.root)
5 val mapSurface = binding.gemSurface
6 mapSurface.onDefaultMapViewCreated = { mapView ->
7 val predefinedPlaces = arrayListOf(
8 Pair("Subway", Coordinates(45.75242654325917, 4.828547972110576)),
9 Pair("McDonald's", Coordinates(45.75291679094701, 4.828855627148713)),
10 Pair("Two Amigos", Coordinates(45.75295718457783, 4.828377481057234)),
11 Pair("Le Jardin de Chine", Coordinates(45.75272771410631, 4.828376649181688)),
12 )
13 /* Image and text */
14 val imageTextsCollection = MarkerCollection(EMarkerType.Point, "Restaurants Nearby")
15 for (place in predefinedPlaces) {
16 Marker().apply {
17 setCoordinates(arrayListOf(place.second))
18 name = place.first
19 imageTextsCollection.add(this)
20 }
21 }
22 val image = getBitmap(R.drawable.ic_restaurant_foreground)?.let {
23 Image.produceWithDataBuffer(DataBuffer(toPngByteArray(it)), EImageFileFormat.Png)
24 }
25 val imageTextsSettings = MarkerCollectionRenderSettings(image)
26 imageTextsSettings.labelTextSize = 2.0 //mm
27 imageTextsSettings.labelingMode = EMarkerLabelingMode.Item
28 mapView.preferences?.markers?.add(imageTextsCollection, imageTextsSettings)
29 /* Polyline */
30 val polylineCollection = MarkerCollection(EMarkerType.Polyline, "Polyline")
31 Marker().apply {
32 for (place in predefinedPlaces)
33 add(place.second)
34 polylineCollection.add(this)
35 }
36 val polylineSettings = MarkerCollectionRenderSettings(polylineInnerColor = Rgba.blue())
37 polylineSettings.polylineInnerSize = 1.5 //mm
38 mapView.preferences?.markers?.add(polylineCollection, polylineSettings)
39 /* Center map on result */
40 mapView.centerOnCoordinates(predefinedPlaces[0].second, 80)
41 }
42 if (!Util.isInternetConnected(this)) {
43 Toast.makeText(this, "You must be connected to internet!", Toast.LENGTH_LONG).show()
44 }
45}
MainActivity
overrides the
onCreate()
function which checks that
internet access is available, and then, when the map is instantiated, defines
an array containing 4 POI (points of interest - restaurants in this case)
coordinate pairs - name, latitude, longitude:
mapSurface.onDefaultMapViewCreated
=
{
mapView
->
val
predefinedPlaces
=
arrayListOf(
val
imageTextsCollection
=
MarkerCollection(EMarkerType.Point,
"Restaurants
Nearby")
for
(place
in
predefinedPlaces)
{
val
polylineCollection
=
MarkerCollection(EMarkerType.Polyline,
"Polyline")
Marker().apply
{
for
(place
in
predefinedPlaces)
val
polylineSettings
=
MarkerCollectionRenderSettings(polylineInnerColor
=
Rgba.blue())
polylineSettings.polylineInnerSize
=
1.5
//mm
mapView.centerOnCoordinates(predefinedPlaces[0].second,
80)