Fly To Route Instruction ¶
Setup ¶
First, get an API key token, see the Getting Started guide.
Download the Maps & Navigation SDK for Android archive file
Download the
FlyToRouteInstruction
project
archive file or clone the project with Git
See the Configure Android Example guide.
Run the example ¶
In Android Studio, from the
File
menu, select
Sync
Project
with
Gradle
Files
|
|||
How it works ¶
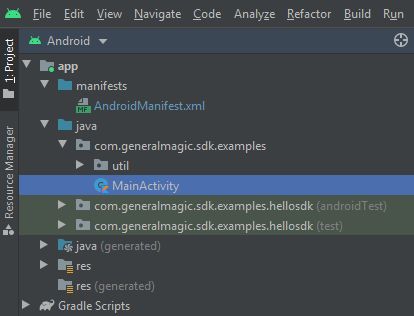
You can open the MainActivity.kt file to see how to fly to a specific instruction along a computed route on the map.
1private val routingService = RoutingService(
2 onStarted = {
3 progressBar.visibility = View.VISIBLE
4 },
5 onCompleted = onCompleted@{ routes, gemError, _ ->
6 progressBar.visibility = View.GONE
7 when (gemError) {
8 GemError.NoError -> {
9 if (routes.size == 0) return@onCompleted
10 // Get the main route from the ones that were found.
11 val route = routes[0]
12 SdkCall.execute {
13 val instructions = route.instructions
14 if (instructions.size == 0) {
15 showToast("No route instructions found!")
16 return@execute
17 }
18 // Get an instruction from the main route.
19 val instruction = route.instructions.let {
20 if (it.size >= 4) it[3] else it[0]
21 }
22 // Add the main route to the map so it can be displayed.
23 gemSurfaceView.mapView?.presentRoute(route)
24 flyToInstruction(instruction)
25 }
26 }
27 GemError.Cancel -> {
28 // The routing action was canceled.
29 }
30 else -> {
31 // There was a problem at computing the routing operation.
32 showToast("Routing service error: ${GemError.getMessage(gemError)}")
33 }
34 }
35 }
36)
RoutingService
is instantiated to compute a route and render
it on the map. The
onStarted
and
onCompleted
callbacks are
implemented, to detect when the route computation is started and when
it is completed.
routes[]
array
of possible routes, then the first route is selected, (at index 0):
val
route
=
routes[0]
if
(it.size
>=
4)
it[3]
else
it[0]
gemSurfaceView.mapView?.presentRoute(route)
flyToInstruction(instruction)
1private fun flyToInstruction(instruction: RouteInstruction) = SdkCall.execute {
2 // Center the map on a specific route instruction using the provided animation.
3 gemSurfaceView.mapView?.centerOnRouteInstruction(instruction)
4}
flyToInstruction()
function is implemented using
gemSurfaceView.mapView?.centerOnRouteInstruction(instruction)
.
1override fun onCreate(savedInstanceState: Bundle?) {
2 super.onCreate(savedInstanceState)
3 setContentView(R.layout.activity_main)
4 progressBar = findViewById(R.id.progressBar)
5 gemSurfaceView = findViewById(R.id.gem_surface)
6 SdkSettings.onMapDataReady = onMapDataReady@{ isReady ->
7 if (!isReady) return@onMapDataReady
8 SdkCall.execute {
9 val waypoints = arrayListOf(
10 Landmark("London", 51.5073204, -0.1276475),
11 Landmark("Paris", 48.8566932, 2.3514616)
12 )
13 routingService.calculateRoute(waypoints)
14 }
15 }
16 SdkSettings.onApiTokenRejected = {
17 showToast("TOKEN REJECTED")
18 }
19 if (!Util.isInternetConnected(this)) {
20 Toast.makeText(this, "You must be connected to internet!",
21 Toast.LENGTH_LONG).show()
22 }
23}
MainActivity
overrides the
onCreate()
function which checks
that internet access is available,
and then, when the map is initialized and ready, defines two waypoints,
and uses the routing service to calculate a route.
onCompleted
callback
flies the camera to the 4th, or if not available, the first instruction,
of the first route in the resulting list of routes.