Magic Lane Documentation
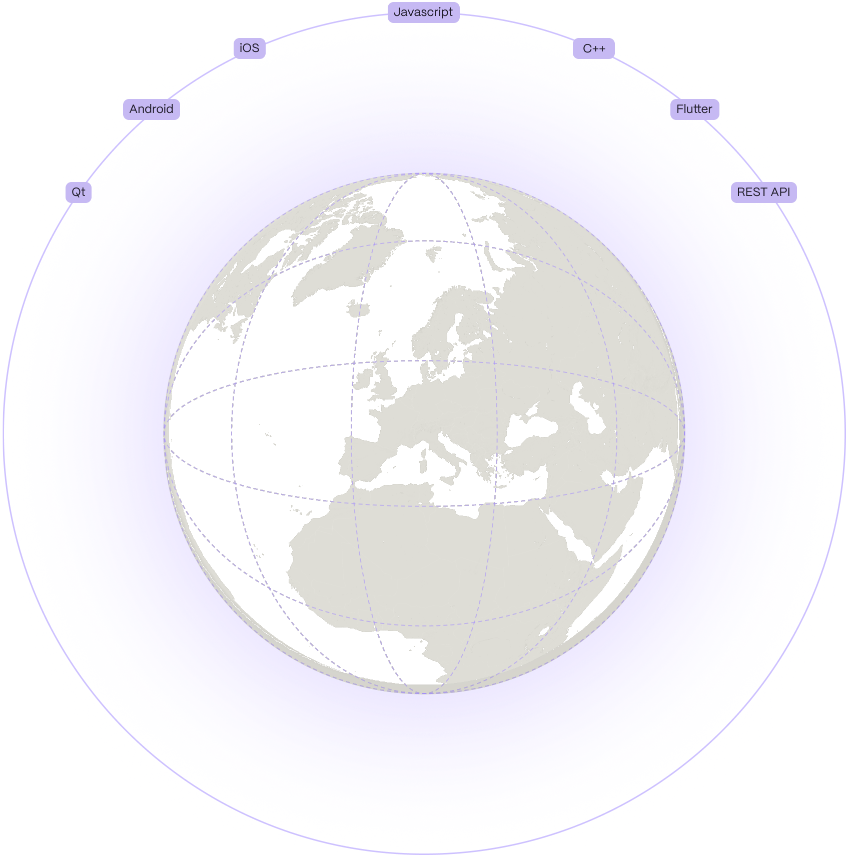
Maps client SDKs
Maps SDK for Javascript
Discover how to integrate search functionality, build routes, and enable navigation on custom interactive maps within your web application.
Maps SDK for Android
Build interactive Android maps with the Maps SDK, featuring 3D views, navigation, styling, offline support, and real-time location tracking.
Maps SDK for IOS
Develop immersive iOS maps with 3D views, navigation, offline access, and real-time tracking.
Maps SDK for Flutter
Create powerful Flutter apps with global maps, offline routing, and optimized navigation for cars, pedestrians, and cyclists.
REST APIs
Fleet Management
Streamline fleet operations with VRP optimization for efficient route planning, order fulfillment, and vehicle assignments, reducing costs and maximizing productivity.
Maps
A stateless, reliable Maps REST API for global coverage, real-time navigation, offline maps, and terrain profiling.